Reimplement the ArrayStack class, f
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 1TF
Related questions
Question
Java
help wih R 7.10:
R-7.10 Reimplement the ArrayStack class, from Section 6.1.2, using dynamic arrays to support unlimited capacity.
![6.1.2 A Simple Array-Based Stack Implementation
As our first implementation of the stack ADT, we store elements in an array, named
data, with capacity N for some fixed N. We oriented the stack so that the bottom
element of the stack is always stored in cell data [0], and the top element of the
stack in cell data[t] for index t that is equal to one less than the current size of the
stack. (See Figure 6.2.)
12345678
9
10
11
12
13
14
15
16
17
18
1 public class ArrayStack<E> implements Stack<E> {
public static final int CAPACITY=1000;
private E[] data;
private int t = −1;
public ArrayStack() { this(CAPACITY);
public ArrayStack(int capacity) {
data (E[]) new Object[capacity];
PATEEN385
20
21
data: A | B
0
1
2
t
N-1
Figure 6.2: Representing a stack with an array; the top element is in cell data[t].
Recalling that arrays start at index 0 in Java, when the stack holds elements
from data [0] to data[t] inclusive, it has size t + 1. By convention, when the stack is
empty it will have t equal to −1 (and thus has size t + 1, which is 0). A complete
Java implementation based on this strategy is given in Code Fragment 6.2 (with
Javadoc comments omitted due to space considerations).
23
}
19 public E pop() {
24
25
=
26 }
==
22 data[t] = null;
t--;
return answer;
CDEFG
}
}
public int size() { return (t + 1); }
public boolean isEmpty() { return (t == -1); }
public void push(E e) throws IllegalStateException {
if (size()
data[++t] = e;
}
public E top() {
if (isEmpty()) return null;
return data[t];
KLM
data.length) throw new IllegalState Exception("Stack is full");
// increment t before storing new item
if (isEmpty()) return null;
E answer = data[t];
// default array capacity
// generic array used for storage
// index of the top element in stack
} // constructs stack with default capacity
// constructs stack with given capacity
// safe cast; compiler may give warning
// dereference to help garbage collection
Code Fragment 6.2: Array-based implementation of the Stack interface.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe8f98c03-2dea-45fe-9e7d-c001811b98bd%2F9b760ee3-7fa4-44a4-80b1-87e841398ea6%2Fvierozj_processed.png&w=3840&q=75)
Transcribed Image Text:6.1.2 A Simple Array-Based Stack Implementation
As our first implementation of the stack ADT, we store elements in an array, named
data, with capacity N for some fixed N. We oriented the stack so that the bottom
element of the stack is always stored in cell data [0], and the top element of the
stack in cell data[t] for index t that is equal to one less than the current size of the
stack. (See Figure 6.2.)
12345678
9
10
11
12
13
14
15
16
17
18
1 public class ArrayStack<E> implements Stack<E> {
public static final int CAPACITY=1000;
private E[] data;
private int t = −1;
public ArrayStack() { this(CAPACITY);
public ArrayStack(int capacity) {
data (E[]) new Object[capacity];
PATEEN385
20
21
data: A | B
0
1
2
t
N-1
Figure 6.2: Representing a stack with an array; the top element is in cell data[t].
Recalling that arrays start at index 0 in Java, when the stack holds elements
from data [0] to data[t] inclusive, it has size t + 1. By convention, when the stack is
empty it will have t equal to −1 (and thus has size t + 1, which is 0). A complete
Java implementation based on this strategy is given in Code Fragment 6.2 (with
Javadoc comments omitted due to space considerations).
23
}
19 public E pop() {
24
25
=
26 }
==
22 data[t] = null;
t--;
return answer;
CDEFG
}
}
public int size() { return (t + 1); }
public boolean isEmpty() { return (t == -1); }
public void push(E e) throws IllegalStateException {
if (size()
data[++t] = e;
}
public E top() {
if (isEmpty()) return null;
return data[t];
KLM
data.length) throw new IllegalState Exception("Stack is full");
// increment t before storing new item
if (isEmpty()) return null;
E answer = data[t];
// default array capacity
// generic array used for storage
// index of the top element in stack
} // constructs stack with default capacity
// constructs stack with given capacity
// safe cast; compiler may give warning
// dereference to help garbage collection
Code Fragment 6.2: Array-based implementation of the Stack interface.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
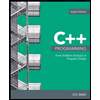
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
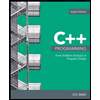
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning