rotate_quadrants
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In puthon

Transcribed Image Text:Next, you will be implementing the rotate_quadrants
function, in which we will split the image into four equal
sized quadrants, and rotate them clockwise.
12
1.
8
2₁
8
(0, 2)
(0, 6)
6.
2
(3, 2)
→
You may assume that the height and width of the image
are even numbers, so that the image can be evenly
divided into quadrants.
Upper left quadrant: (x, y)
• Upper right quadrant: (x + w/2, y)
Lower right quadrant: (x + w/2, y +h/2)
● Lower left quadrant: (x, y + h/2)
Hint:
While you could break this up into four separate matrices,
it's probably easier to think of this on a pixel-by-pixel
basis. In particular, if the picture has height h and width
w, and a pixel is at position (x, y) in the upper left
quadrant, then the four pixels it needs to rotate with are:
(3,6)
20
8
6.
=
For example, in the image below, width = 6 and height :
8. So the pixel at (0, 2) would rotate with the pixels at
(0+3, 2), (0+3, 2+4), and (0, 2+4). That is: (3, 2), (3, 6),
and (0, 6).
(0, 2)
8
(0, 6)
12
8
(3, 2)
(3,6)
![>>> rotate_quadrants([[[127, 127, 127],
[0, 0, 0]],
[[255, 255, 0], [50, 128, 255]],
[[0, 0, 255], [0, 255, 0]],
[[255, 0, 0], [255, 255, 255]]])
[[[0, 0, 255], [127, 127, 127]],
[[255, 0, 0], [255, 255, 0]],
[[0, 255, 0], [0, 0, 0]],
[[255, 255, 255], [50, 128, 255]]]
>>> rotate_quadrants(
[[ [255, 0, 0], [255,153,0],
[255,255,0],[255,204,51]],
[[0, 255, 0],
[0,255,255], [50, 128, 255], [255,204,51]],
[ [0, 0, 255], [153,0,255],
[255,0,255], [255, 204,51]],
[ [0, 0, 0], [255, 204,51], [122,0,25],
[122,0,25]],
[[255, 204,51], [122,0,25], [122,0,25],
[122,0,25] ],
[ [122,0,25], [122,0,25], [122,0,25],
[122,0,25]],
[ [122,0,25], [122,0,25], [122,0,25],
[122,0,25]],
[ [122,0,25], [122,0,25], [122,0,25],
[122,0,25] ]])
[[[255, 204,51], [122,0,25], [255, 0, 0],
[255,153,0]],
[ [122,0,25],
[122,0,25], [0, 255, 0],
[0, 255, 255]],
[ [122,0,25], [122,0,25], [0, 0, 255],
[153,0,255]],
[ [122,0,25], [122,0,25],
0], [255, 204,51]],
[ [122,0,25], [122,0,25] |
[255,255,0], [255, 204,51]],
[ [122,0,25], [122,0,25]
,[50, 128, 255], [255, 204,51]],
[ [122,0,25], [122,0,25],
[255,0,255], [255, 204,51]],
[ [122,0,25], [122,0,25], [122,0,25],
[122,0,25] ]]
[0, 0,](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fea258185-b79a-47d1-99df-8ec857b3195a%2Fa91e31e8-ad8e-46fa-90b2-51d7ee617470%2Fd7s7tqo_processed.jpeg&w=3840&q=75)
Transcribed Image Text:>>> rotate_quadrants([[[127, 127, 127],
[0, 0, 0]],
[[255, 255, 0], [50, 128, 255]],
[[0, 0, 255], [0, 255, 0]],
[[255, 0, 0], [255, 255, 255]]])
[[[0, 0, 255], [127, 127, 127]],
[[255, 0, 0], [255, 255, 0]],
[[0, 255, 0], [0, 0, 0]],
[[255, 255, 255], [50, 128, 255]]]
>>> rotate_quadrants(
[[ [255, 0, 0], [255,153,0],
[255,255,0],[255,204,51]],
[[0, 255, 0],
[0,255,255], [50, 128, 255], [255,204,51]],
[ [0, 0, 255], [153,0,255],
[255,0,255], [255, 204,51]],
[ [0, 0, 0], [255, 204,51], [122,0,25],
[122,0,25]],
[[255, 204,51], [122,0,25], [122,0,25],
[122,0,25] ],
[ [122,0,25], [122,0,25], [122,0,25],
[122,0,25]],
[ [122,0,25], [122,0,25], [122,0,25],
[122,0,25]],
[ [122,0,25], [122,0,25], [122,0,25],
[122,0,25] ]])
[[[255, 204,51], [122,0,25], [255, 0, 0],
[255,153,0]],
[ [122,0,25],
[122,0,25], [0, 255, 0],
[0, 255, 255]],
[ [122,0,25], [122,0,25], [0, 0, 255],
[153,0,255]],
[ [122,0,25], [122,0,25],
0], [255, 204,51]],
[ [122,0,25], [122,0,25] |
[255,255,0], [255, 204,51]],
[ [122,0,25], [122,0,25]
,[50, 128, 255], [255, 204,51]],
[ [122,0,25], [122,0,25],
[255,0,255], [255, 204,51]],
[ [122,0,25], [122,0,25], [122,0,25],
[122,0,25] ]]
[0, 0,
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
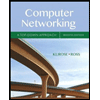
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
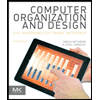
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
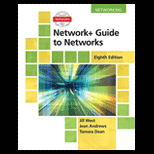
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
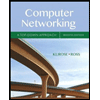
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
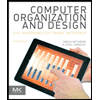
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
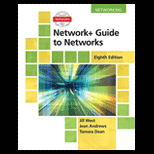
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
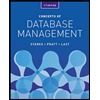
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
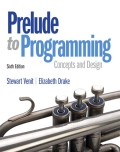
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
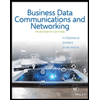
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY