Salary Calculator Create a program that computes the salary based on an employee's hourly wage and hours worked. Note that employees may work for fractions of an hour, e.g. 1 and a half hours (1.5 hours). Use the following formulas: Less than or equal to 40 hours worked hourly wage * hours worked Over 40, but less than or equal to 65 hours worked (hourly wage * 40) + (hours worked - 40) * (hourly wage * 1.5) Over 65 hours worked (hourly wage * 40) + (hourly wage * 1.5) * 25 + (hours worked - 65) * hourly wage * 2 Also make sure that the hourly wage and hours worked are positive. Otherwise, display the error message: "Invalid input". Please see the sample outputs below to guide the design of your program. main.cc file #include #include int main() { // TODO: accept user input to store the hourly wage and hours worked. // Then, include the header file at the top of this file so you can // call your function that computes a salary based on these inputs. // Don't forget to check for invalid inputs! return0; } salary.cc file // TODO: implement the function defined in salary.h here. salary.h file // TODO: write your function declaration here.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Salary Calculator
Create a
Less than or equal to 40 hours worked
hourly wage * hours worked
Over 40, but less than or equal to 65 hours worked
(hourly wage * 40) + (hours worked - 40) * (hourly wage * 1.5)
Over 65 hours worked
(hourly wage * 40) + (hourly wage * 1.5) * 25 + (hours worked - 65) * hourly wage * 2
Also make sure that the hourly wage and hours worked are positive. Otherwise, display the error message: "Invalid input".
Please see the sample outputs below to guide the design of your program.
main.cc file
#include <iomanip> | |
#include <iostream> | |
int main() { | |
// TODO: accept user input to store the hourly wage and hours worked. | |
// Then, include the header file at the top of this file so you can | |
// call your function that computes a salary based on these inputs. | |
// Don't forget to check for invalid inputs! | |
return0; | |
} |
salary.cc file
// TODO: implement the function defined in salary.h here.
salary.h file
// TODO: write your function declaration here.
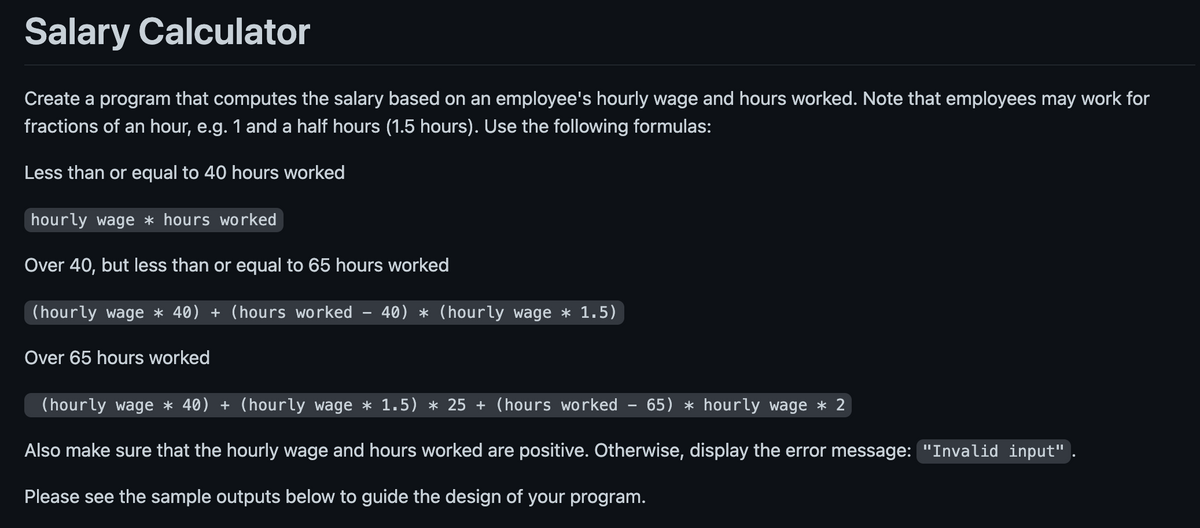
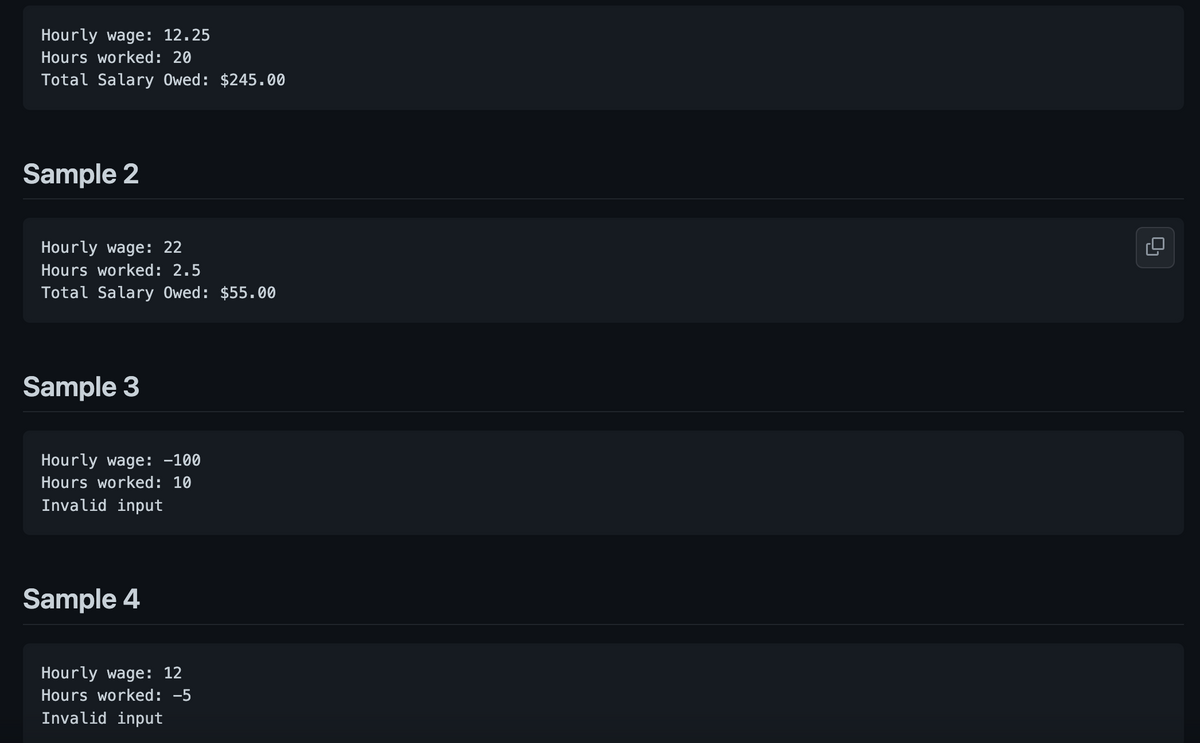

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

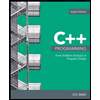
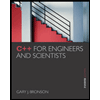
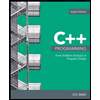
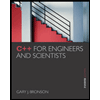