Step 1: Preparation Comment out the following under-construction code: In dynamicaray.h: Al function prototypes except the constructors and destructor. Keep the member variables. In dynamicarray.cpp: All function implementations except the constructors and destructor. Also remove (not just comment out) INITIAL_CAP, and replace it with a hard-coded 10 inside the default constructor. This will also eventually go away. In main: Comment out all the code inside the RunPart1Tests function between the lines. Also in main: Comment out all the code in the main function starting with the "Equality comparison" comment and just before the "return O;" line. bool pass = true; and return pass; Step 2: Replacing member data and the two constructors Replace the current member data (arr, len, and capacity) with a single vector of integers. At the top of your h, include the vector library. This is the only place you should need to include it. Also in your .h, remove your current member data, replace it with a vector of ints. Name it "arr" so you can keep as much of your current code as possible. Next, you need to change the constructor and destructor. For the copy constructor, copy the vector from the other object; do so by doing an element-by-element copy. Here's your new copy constructor in all its glory: DynamicArray::DynamicArray(const DynamicArray& other) : arr(other.arr) {} It should report the Lab 6 tests as "passed." Step 3: The one-liners plus output Tackle the four member functions (cap(), at), append() and operator=) that translate very well to functions that the vector class provides for you. You'll also tackle print, since you'll need it to check your results. What to Do: Uncomment the declarations and definitions from the .h and .cpp files Replace cap() function (defined in the .h) with a single-line function that uses a member function of the vector class. Do the same for the at() and append() function. Do not return -11111 when out of bound Repeat for the = operator. This will be two lines: one to actually copy the vector. and then the obligatory return * this; Replace these references with the appropriate ways to access them using your new vector. When you're ready to test your code, go to the RunPart1 Tests function in main.cpp. Put everything back in until the line labeled "Test sum." Two bad things are going to hapen: You're going to fail two capacity tests. You can comment out the sections that test the capacity. This code should crash, with an error message including: terminate called after throwing an instance of 'std::out_of_range: vector'
Step 1: Preparation
Comment out the following under-construction code:
- In dynamicaray.h: Al function prototypes except the constructors and destructor. Keep the member variables.
- In dynamicarray.cpp: All function implementations except the constructors and destructor. Also remove (not just comment out) INITIAL_CAP, and replace it with a hard-coded 10 inside the default constructor. This will also eventually go away.
- In main: Comment out all the code inside the RunPart1Tests function between the lines.
Also in main: Comment out all the code in the main function starting with the "Equality comparison" comment and just before the "return O;" line.
bool pass = true;
and
return pass;
Step 2: Replacing member data and the two constructors
Replace the current member data (arr, len, and capacity) with a single
- At the top of your h, include the vector library. This is the only place you should need to include it.
- Also in your .h, remove your current member data, replace it with a vector of ints. Name it "arr" so you can keep as much of your current code as possible.
Next, you need to change the constructor and destructor.
- For the copy constructor, copy the vector from the other object; do so by doing an element-by-element copy.
Here's your new copy constructor in all its glory:
DynamicArray::DynamicArray(const DynamicArray& other) : arr(other.arr) {}
It should report the Lab 6 tests as "passed."
Step 3: The one-liners plus output
Tackle the four member functions (cap(), at), append() and operator=) that translate very well to functions that the vector class provides for you. You'll also tackle print, since you'll need it to check your results.
What to Do:
- Uncomment the declarations and definitions from the .h and .cpp files
- Replace cap() function (defined in the .h) with a single-line function that uses a member function of the vector class.
- Do the same for the at() and append() function. Do not return -11111 when out of bound
- Repeat for the = operator. This will be two lines: one to actually copy the vector. and then the obligatory return * this;
Replace these references with the appropriate ways to access them using your new vector.
When you're ready to test your code, go to the RunPart1 Tests function in main.cpp. Put everything back in until the line labeled "Test sum."
Two bad things are going to hapen:
- You're going to fail two capacity tests. You can comment out the sections that test the capacity.
- This code should crash, with an error message including:
terminate called after throwing an instance of 'std::out_of_range: vector'


Step by step
Solved in 4 steps with 5 images

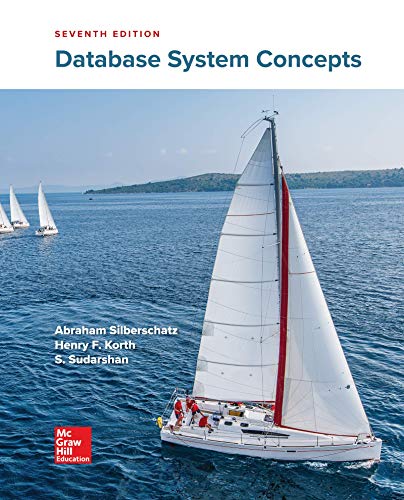
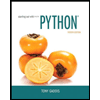
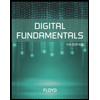
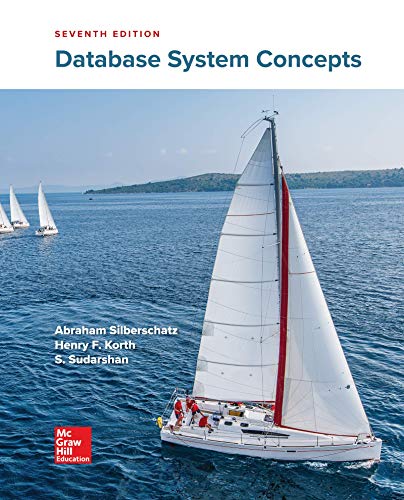
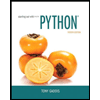
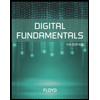
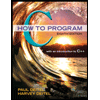
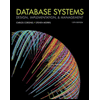
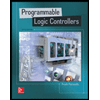