Step 1: Write a search function called linkedlistSearch( ) that takes a number, traverse through a linked list and return true if the number exists it the list and false otherwise. Running the code should look like: Enter list numbers separated by space, followed by -1: 3 5 7 -1 Menu options: 1. Search for a value. 2. Display the sum of all numbers on the list. 3. Exit program. Your choice -> 1 Enter a value to initiate a search: 4 The number you entered does not exist in this list.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
C++
Given code
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node *pNext;
};
void displayNumberValues( Node *pHead)
{
while( pHead != NULL) {
cout << pHead->data << " ";
pHead = pHead->pNext;
}
cout << endl;
}
//Option 1: Search the list
// TODO: complete the function below to search for a given value in linked lsit
// return true if value exists in the list, return false otherwise.
?? linkedlistSearch( ???)
{
}
//Option 2: get sum of all values
// TODO: complete the function below to return the sum of all elements in the linked list.
??? getSumOfAllNumbers( ???)
{
}
int main()
{
int userInput;
Node *pHead = NULL;
Node *pTemp;
cout<<"Enter list numbers separated by space, followed by -1: ";
cin >> userInput;
// Keep looping until end of input flag of -1 is given
while( userInput != -1) {
// Store this number on the list
pTemp = new Node;
pTemp->data = userInput;
pTemp->pNext = pHead;
pHead = pTemp;
cin >> userInput;
}
cout <<" Menu options:\n";
cout <<" 1. Search for a value. \n"
<<" 2. Display the sum of all numbers on the list. \n"
<<" 3. Exit program. \n";
cin >> userInput;
cout<<"Your choice ->" << userInput <<endl;
switch(userInput){
case(1):
int num;
cout<<"Enter a value to initiate a search:"<<endl;
cin >> num;
//Call search function here
//Display results
break;
case(2):
// Call getSumOfAllNumbers here
//Display results
break;
case(3):
exit(0);
}
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

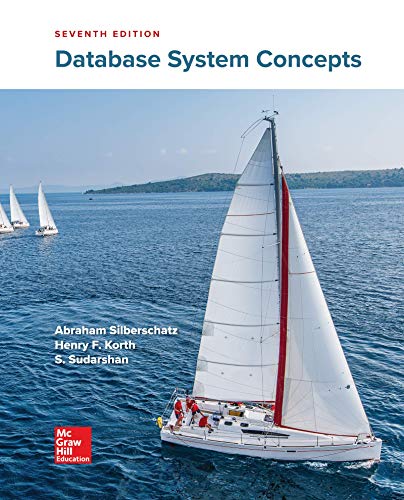
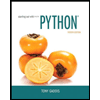
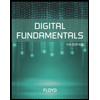
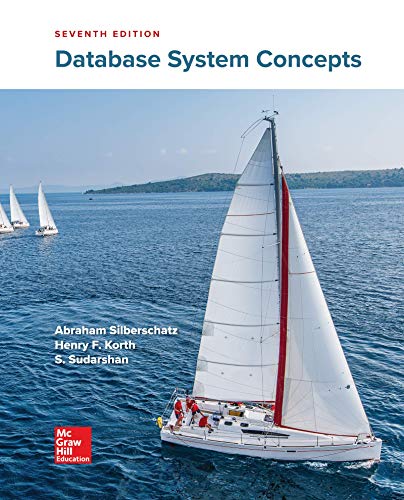
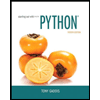
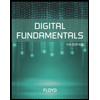
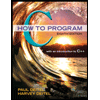
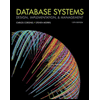
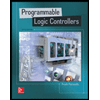