TCP connection-Server and Client connection using C. Please adjust the given code as needed. Do not change to another language. Please provide screenshots of it's execution. 1.- client read a line of characters(data) from its keyboard and sends the data to the server. 2- the server receives the data and converts characters to uppercase. 3- the server sends the modified data to the client. 4- the client receives the modified data and displays the line on its screen.
TCP connection-Server and Client connection using C. Please adjust the given code as needed. Do not change to another language. Please provide screenshots of it's execution.
1.- client read a line of characters(data) from its keyboard and sends the data to the server.
2- the server receives the data and converts characters to uppercase.
3- the server sends the modified data to the client.
4- the client receives the modified data and displays the line on its screen.
//Server.c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <arpa/inet.h>
#include <sys/socket.h>
// Add header files if needed
void error_handling(char *message);
int main(int argc, char *argv[])
{
int serv_sock;
int clnt_sock;
struct sockaddr_in serv_addr;
struct sockaddr_in clnt_addr;
socklen_t clnt_addr_size;
// Declarer variables
char message[]="Hello Socket Programming!";
if(argc!=2){
printf("Usage : %s <port>\n", argv[0]);
exit(1);
}
serv_sock=socket(PF_INET, SOCK_STREAM, 0);
if(serv_sock == -1)
error_handling("socket() error");
memset(&serv_addr, 0, sizeof(serv_addr));
serv_addr.sin_family=AF_INET;
serv_addr.sin_addr.s_addr=htonl(INADDR_ANY);
serv_addr.sin_port=htons(atoi(argv[1]));
if(bind(serv_sock, (struct sockaddr*) &serv_addr, sizeof(serv_addr))==-1 )
error_handling("bind() error");
if(listen(serv_sock, 5)==-1)
error_handling("listen() error");
clnt_addr_size=sizeof(clnt_addr);
clnt_sock=accept(serv_sock, (struct sockaddr*)&clnt_addr,&clnt_addr_size);
if(clnt_sock==-1)
error_handling("accept() error");
while(1){
// write(clnt_sock, message, sizeof(message));
// Implement code
// 1. Received messages from the connected client
// 2. Change all lower case letters to their upper cases
// 3. Return the modified data back to the client
}
close(clnt_sock);
close(serv_sock);
return 0;
}
void error_handling(char *message)
{
fputs(message, stderr);
fputc('\n', stderr);
exit(1);
}
//Client.c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <arpa/inet.h>
#include <sys/socket.h>
// Add header files
void error_handling(char *message);
int main(int argc, char* argv[])
{
int sock;
struct sockaddr_in serv_addr;
char message[30];
int str_len;
// Declarer variables
if(argc!=3){
printf("Usage : %s <IP> <port>\n", argv[0]);
exit(1);
}
sock=socket(PF_INET, SOCK_STREAM, 0);
if(sock == -1)
error_handling("socket() error");
memset(&serv_addr, 0, sizeof(serv_addr));
serv_addr.sin_family=AF_INET;
serv_addr.sin_addr.s_addr=inet_addr(argv[1]);
serv_addr.sin_port=htons(atoi(argv[2]));
if(connect(sock, (struct sockaddr*)&serv_addr, sizeof(serv_addr))==-1)
error_handling("connect() error!");
while(1){
/*
str_len=read(sock, message, sizeof(message)-1);
if(str_len==-1)
error_handling("read() error!");
printf("Message from server: %s \n", message);
*/
// Implement the code
// 1. Take input from the keyboard as a string
// 2. Send the message from the keyboard
// 3. Wait and receive a message from the connected server
// 4. Print out the received message on the terminal
}
close(sock);
return 0;
}
void error_handling(char *message)
{
fputs(message, stderr);
fputc('\n', stderr);
exit(1);
}

Step by step
Solved in 3 steps with 1 images

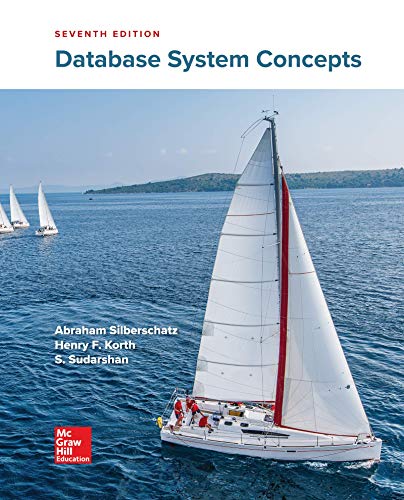
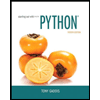
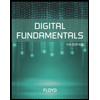
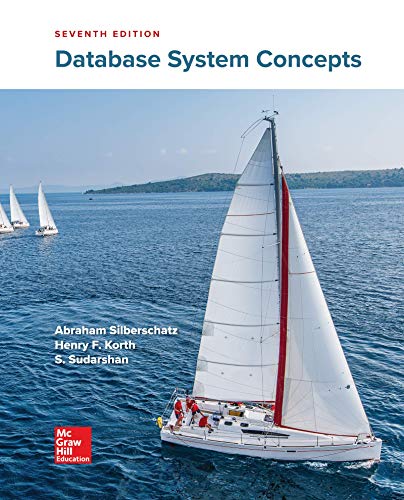
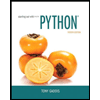
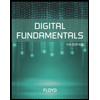
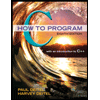
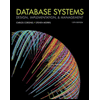
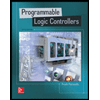