Given the c language below, I am lost why the outpout shows random characters when I want them to be words. Could you help me improve my code, please thank you. A picture shows the error output. #include #include #include int main () { char string[1000], word[1000], array[100][150]; //showing the maximum size for string, word and array int i = 0, j = 0, k = 0, len1 = 0, len2 = 0, count = 0; printf ("Enter the string:\n"); //user input a sentence gets(string); printf ("Enter the word to be removed:\n"); //inputs the words to be removed gets (word); // (Removing process condition) for (i = 0; string[i] != '\0'; i++) { if (string[i] == ' ') { array[k][j] = '\0'; k ++; j = 0; } else { array[k][j] = array[i]; j ++; } } array[k][j] = '\0'; j = 0; for (i = 0; i < k + 1; i++) { if (strcmp(array[i], word) == 0) { array[i][j] = '\0'; } } j = 0; // calculate words before removing printf("The words before removing: "); // Returns first token char* token = strtok(string, " "); // always keep token's printing while (token != NULL) { count+=1; token = strtok(NULL, " "); } // if word has any break it will count +1 printf("%d\n", count); count = 0; // printing the sentence after removing printf ("String or sentence after removing: ", word); for (i = 0; i < k + 1; i++) { if (array[i][j] == '\0') continue; else { printf ("%s ", array[i]); count++; } } printf ("\n"); //calculating words after removing of letter printf("The words after removing: "); printf("%d\n", count); return 0; }
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
Given the c language below, I am lost why the outpout shows random characters when I want them to be words. Could you help me improve my code, please thank you. A picture shows the error output.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main ()
{
char string[1000], word[1000], array[100][150]; //showing the maximum size for string, word and array
int i = 0, j = 0, k = 0, len1 = 0, len2 = 0, count = 0;
printf ("Enter the string:\n");
//user input a sentence
gets(string);
printf ("Enter the word to be removed:\n");
//inputs the words to be removed
gets (word);
// (Removing process condition)
for (i = 0; string[i] != '\0'; i++)
{
if (string[i] == ' ')
{
array[k][j] = '\0';
k ++;
j = 0;
}
else
{
array[k][j] = array[i];
j ++;
}
}
array[k][j] = '\0';
j = 0;
for (i = 0; i < k + 1; i++)
{
if (strcmp(array[i], word) == 0)
{
array[i][j] = '\0';
}
}
j = 0;
// calculate words before removing
printf("The words before removing: ");
// Returns first token
char* token = strtok(string, " ");
// always keep token's printing
while (token != NULL) {
count+=1;
token = strtok(NULL, " ");
}
// if word has any break it will count +1
printf("%d\n", count);
count = 0;
// printing the sentence after removing
printf ("String or sentence after removing: ", word);
for (i = 0; i < k + 1; i++)
{
if (array[i][j] == '\0')
continue;
else
{
printf ("%s ", array[i]);
count++;
}
}
printf ("\n");
//calculating words after removing of letter
printf("The words after removing: ");
printf("%d\n", count);
return 0;
}


Step by step
Solved in 2 steps with 2 images

The code is here for C language. But the words did not get remove as wanted. The output picture shows the output. Please help.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main ()
{
char string[1000], word[1000], array[100][150]; //showing the maximum size for string, word and array
int i = 0, j = 0, k = 0, len1 = 0, len2 = 0, count = 0;
printf ("Enter the string:\n");
//user input a sentence
gets(string);
printf ("Enter the word to be removed:\n");
//inputs the words to be removed
gets (word);
// (Removing process condition)
for (i = 0; string[i] != '\0'; i++)
{
if (string[i] == ' ')
{
array[k][j] = '\0';
k ++;
j = 0;
}
else
{
array[k][j] = string[i]; // Change 1
j ++;
}
}
array[k][j] = '\0';
j = 0;
for (i = 0; i < k + 1; i++)
{
if (strcmp(array[i], word) == 0)
{
array[i][j] = '\0';
}
}
j = 0;
// calculate words before removing
printf("The words before removing: ");
// Returns first token
char* token = strtok(string, " ");
// always keep token's printing
while (token != NULL) {
count+=1;
token = strtok(NULL, " ");
}
// if word has any break it will count +1
printf("%d\n", count);
count = 0;
// printing the sentence after removing
printf ("String or sentence after removing: ", word);
for (i = 0; i < k + 1; i++)
{
if (array[i][j] == '\0')
continue;
else
{
printf ("%s ", array[i]);
count++;
}
}
printf ("\n");
//calculating words after removing of letter
printf("The words after removing: ");
printf("%d\n", count);
return 0;
}
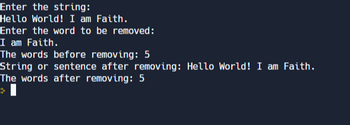
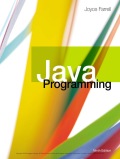
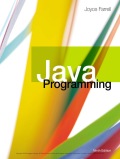