URGENT!!!! Please use this graph class and SOLVE FOR ISOLATEDTRIPLETS QUESTION AND AVERAGE BALANCE RATIO Here is the graph class: public class Graph { private final int V; private int E; private final Bag[] adj; /** * Create an empty graph with V vertices. */ @SuppressWarnings("unchecked") public Graph(int V) { if (V < 0) thr
(0)
URGENT!!!!
Please use this graph class and SOLVE FOR ISOLATEDTRIPLETS QUESTION AND AVERAGE BALANCE RATIO
Here is the graph class:
public class Graph {
private final int V;
private int E;
private final Bag<Integer>[] adj;
/**
* Create an empty graph with V vertices.
*/
@SuppressWarnings("unchecked")
public Graph(int V) {
if (V < 0) throw new Error("Number of vertices must be nonnegative");
this.V = V;
this.E = 0;
this.adj = new Bag[V];
for (int v = 0; v < V; v++) {
adj[v] = new Bag<>();
}
}
/**
* Return the number of vertices in the graph.
*/
public int V() { return V; }
/**
* Return the number of edges in the graph.
*/
public int E() { return E; }
/**
* Add the undirected edge v-w to graph.
* @throws java.lang.IndexOutOfBoundsException unless both 0 <= v < V and 0 <= w < V
*/
public void addEdge(int v, int w) {
if (v < 0 || v >= V) throw new IndexOutOfBoundsException();
if (w < 0 || w >= V) throw new IndexOutOfBoundsException();
E++;
adj[v].add(w);
adj[w].add(v);
}
/**
* Return the list of neighbors of vertex v as in Iterable.
* @throws java.lang.IndexOutOfBoundsException unless 0 <= v < V
*/
public Iterable<Integer> adj(int v) {
if (v < 0 || v >= V) throw new IndexOutOfBoundsException();
return adj[v];
}
/**
* Returns the degree of vertex {@code v}.
*
* @param v the vertex
* @return the degree of vertex {@code v}
* @throws IllegalArgumentException unless {@code 0 <= v < V}
*/
public int degree(int v) {
if (v < 0 || v >= V) throw new IndexOutOfBoundsException();
return adj[v].size();
}
/**
* Return a string representation of the graph.
*/
public String toString() {
StringBuilder s = new StringBuilder();
String NEWLINE = System.getProperty("line.separator");
s.append(V + " vertices, " + E + " edges " + NEWLINE);
for (int v = 0; v < V; v++) {
s.append(v + ": ");
for (int w : adj[v]) {
s.append(w + " ");
}
s.append(NEWLINE);
}
return s.toString();
}
/**
* Save a graphviz representation of the graph.
* See <a href="http://www.graphviz.org/">graphviz.org</a>.
*/
public void toGraphviz(String filename) {
GraphvizBuilder gb = new GraphvizBuilder ();
for (int v = 0; v < V; v++) {
gb.addNode (v);
boolean showSelfLoop = false;
for (int w : adj[v]) {
if (v < w) // only once each edge
gb.addEdge (v, w);
if (v == w) {
showSelfLoop = !showSelfLoop;
if (showSelfLoop)
gb.addEdge (v, w);
}
}
}
gb.toFileUndirected (filename);
}
/**
* Test client.
*/
public static void main(String[] args) {
//args = new String [] { "data/tinyCG.txt" };
args = new String [] { "data/tinyG.txt" };
//args = new String [] { "20", "40" };
Graph G;
if (args.length == 1) {
In in = new In(args[0]);
G = GraphGenerator.fromIn (in);
} else {
int V = Integer.parseInt (args[0]);
int E = Integer.parseInt (args[1]);
G = GraphGenerator.simple(V, E);
}
StdOut.println(G);
G.toGraphviz ("g.png");
}
}
NOW SOLVE FOR
/**
* CountNumberOfIsoTrips
*
* determine how many groups of 3 vertices (u,v,w) form an Isolated Triple -
* that is: 3 vertices directly connected only to each other
* Each group should be counted only one time.
*
* the functions stores the computed result in the numberOfIsoTrips instance variable
*/
private void countNumberOfIsoTrips(Graph G) {
int numofIsoTrio = 0;
}
/**
* balancedFriendships
*
* say the 'balanceRatio' of a friendship is the ratio between the two friends'
* popularities, with the larger popularity as the denominator.
* If two friends have the same popularity, then the balanceRatio would
* be 1. If one of the friends had N total friends and the other had 1, then the
* balanceRatio would be 1/N - it would be a 'lopsided' friendship
*
* the average balanceRatio for a graph is the average of all the balanceRatios for all the edges in the Graph
* (note it would always be >=0)
*
* B-Level:
* determine the average balanceRatio for the graph.
* store the answer in the avgBalanceRatio instance variable
*
* A-Level
*
* determine the number of 'friendships' for which the balanceRatio is greater than the average Balance ratio for the graph
* store the answer in the numberOfAboveAverageFriendships instance variable
*
* this level is optional. if you choose NOT to complete it, simply leave the assignment
* statement to numberOfAboveAverageFriendships as given below.
*
* Example: if all vertices have the same number of friends, then all balanceRatios would be 1
* and so the averageBalanceRatio would be 1
*
* A-Level: since all balanceRatios are the same, none would be aboveAverage
* the numberOfAboveAverageFriendships would be 0
*
* Example: if one vertex 'a' was connected to 5 other vertices (b,c,d,e,f) and b-c-d-e-f made a cycle
* edges, then a's popularity would be 5, all the others would be 3. The balanceRatio for
* all 5 friendships involving 'a' would be 3/5; for the other 5 friendships it would be 1
* so the averageBalanceRatio would 4/5
*
* A-Level: numberOfAboveAverageFriendships would be 5.
*
*/
private void balancedFriendships(Graph G) {
numberOfAboveAverageFriendships = -1; // toDo5-A optional A Level fix this
}
PLEASE HELP
NO USING CHATBOT

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

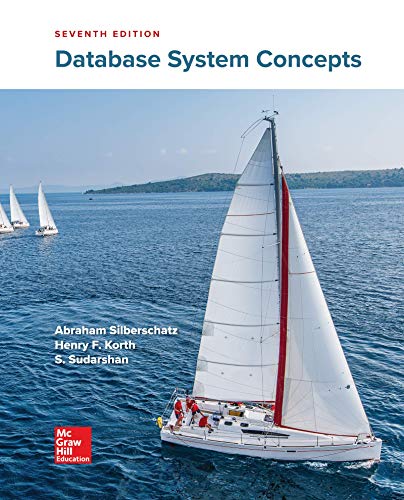
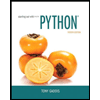
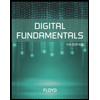
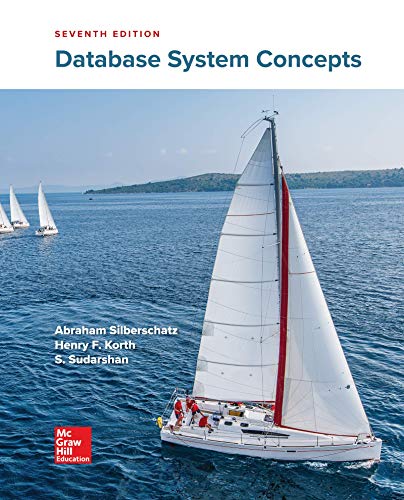
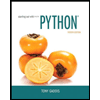
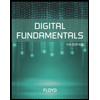
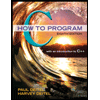
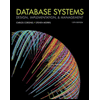
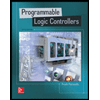