write a function to compute the fewest number of coins that you need to make up that amount.
write a function to compute the fewest number of coins that you need to make up that amount.
Please find the error in my code and explain it with comments
#include <iostream>
using namespace std;
int countChange(int denoms[], int denomsLength, int amount) {
// Edge cases
if( denomsLength < 0)
return 0;
int i, j, x, y;
// We need n+1 rows as the table
// is constructed in bottom up
// manner using the base case 0
// value case (n = 0)
int lookupTable[amount + 1][denomsLength];
// Fill rest of the table entries
// in bottom up manner
for (i = 1; i < amount + 1; i++) {
for (j = 0; j < denomsLength; j++) {
// Count of solutions including denoms[j]
x = (i - denoms[j] >= 0) ? lookupTable[i - denoms[j]][j] : 0;
// Count of solutions excluding denoms[j]
y = (j >= 1) ? lookupTable[i][j - 1] : 0;
// total count
lookupTable[i][j] = y;
}
}
return 0;
}
int main() {
int denoms[4] = {25,10,5,1};
cout << countChange(denoms, 4, 10) << endl;
}

Step by step
Solved in 3 steps with 1 images

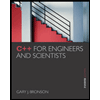
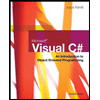
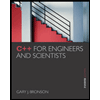
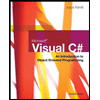