Write in C++ When you're processing data, it’s useful to break up a text string into pieces using a delimiter. Write a function split() that takes a string, splits it at every occurrence of a delimiter, and then populates an array of strings with the split pieces, up to the provided maximum number of pieces. Function specifications: Name: split() Parameters (Your function should accept these parameters IN THIS ORDER): input_string string: The text string containing data separated by a delimiter separator char: The delimiter marking the location where the string should be split up arr string array: The array that will be used to store the input text string's individual string pieces arr_size int: The number of elements that can be stored in the array Return Value: int: The number of pieces the input text string was split into
Write in C++ When you're processing data, it’s useful to break up a text string into pieces using a delimiter. Write a function split() that takes a string, splits it at every occurrence of a delimiter, and then populates an array of strings with the split pieces, up to the provided maximum number of pieces. Function specifications: Name: split() Parameters (Your function should accept these parameters IN THIS ORDER): input_string string: The text string containing data separated by a delimiter separator char: The delimiter marking the location where the string should be split up arr string array: The array that will be used to store the input text string's individual string pieces arr_size int: The number of elements that can be stored in the array Return Value: int: The number of pieces the input text string was split into
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write in C++
When you're processing data, it’s useful to break up a text string into pieces using a delimiter. Write a function split() that takes a string, splits it at every occurrence of a delimiter, and then populates an array of strings with the split pieces, up to the provided maximum number of pieces.
Function specifications:
- Name: split()
- Parameters (Your function should accept these parameters IN THIS ORDER):
- input_string string: The text string containing data separated by a delimiter
- separator char: The delimiter marking the location where the string should be split up
- arr string array: The array that will be used to store the input text string's individual string pieces
- arr_size int: The number of elements that can be stored in the array
- Return Value: int: The number of pieces the input text string was split into
Note:
- No input will have delimiters in the beginning or the end of the string. (Eg: ",apple, orange" OR "apple, orange,")
- No input will have multiple delimiters added consecutively. (Eg: "apple,,,orange,banana")
- If the delimiter character is not found, then the function returns 1 and the entire string is placed in the array as the first element.
- If the string is split into more pieces than the size of the array (the last parameter), then the function returns -1. The array should be filled with as many pieces of the split string as is possible.
- If an empty string is provided then return 0.

Transcribed Image Text:When you're processing data, it's useful to break up a text string into pieces using a delimiter. Write a function split() that takes a string,
splits it at every occurrence of a delimiter, and then populates an array of strings with the split pieces, up to the provided maximum number
of pieces.
Function specifications:
• Name: split()
• Parameters (Your function should accept these parameters IN THIS ORDER):
input_string string: The text string containing data separated by a delimiter
o separator char : The delimiter marking the location where the string should be split up
o arr string array: The array that will be used to store the input text string's individual string pieces
o arr_size int: The number of elements that can be stored in the array
• Return Value: int: The number of pieces the input text string was split into
Note:
O
• No input will have delimiters in the beginning or the end of the string. (Eg: ",apple, orange" OR "apple, orange,")
• No input will have multiple delimiters added consecutively. (Eg: "apple,,,orange, banana")
• If the delimiter character is not found, then the function returns 1 and the entire string is placed in the array as the first element.
• If the string is split into more pieces than the size of the array (the last parameter), then the function returns -1. The array should be
filled with as many pieces of the split string as is possible.
●
If an empty string is provided then return 0.
![Function Call
string test case
char separator
int arr_size = 3;
string arr[size];
// num_splits is the value returned by split
int num_splits =
split(testcase, separator, arr, arr_size);
cout << "Function returned value: " << num_splits << endl;
cout << "arr[0]:"<< arr[0] << endl;
Output
Sample run 2:
"ABCDEFG";
I
Function Call
Function returned value: 1
arr [0] ABCDEFG
;
string testcase = "RST, UVW, XYZ" ;
char separator = ',';
int arr_size = 3;
string arr[size];
// num_splits is the value returned by split
int num_splits
split(testcase, separator, arr, arr_size);
cout << "Function returned value: " << num_splits << endl;
for (int i=0; i < size; i++){
cout << "arr["<< i << "]:" << arr[i] << endl;
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb1d14486-aab8-45fa-9185-3d15b7af8b4b%2F7b533848-d760-4677-99e8-166e28ac453c%2Fq05quyhc_processed.png&w=3840&q=75)
Transcribed Image Text:Function Call
string test case
char separator
int arr_size = 3;
string arr[size];
// num_splits is the value returned by split
int num_splits =
split(testcase, separator, arr, arr_size);
cout << "Function returned value: " << num_splits << endl;
cout << "arr[0]:"<< arr[0] << endl;
Output
Sample run 2:
"ABCDEFG";
I
Function Call
Function returned value: 1
arr [0] ABCDEFG
;
string testcase = "RST, UVW, XYZ" ;
char separator = ',';
int arr_size = 3;
string arr[size];
// num_splits is the value returned by split
int num_splits
split(testcase, separator, arr, arr_size);
cout << "Function returned value: " << num_splits << endl;
for (int i=0; i < size; i++){
cout << "arr["<< i << "]:" << arr[i] << endl;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
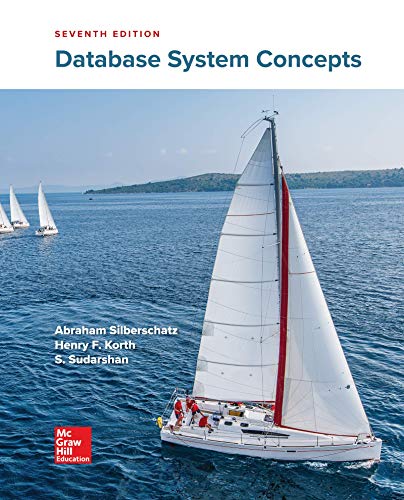
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
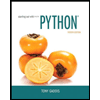
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
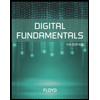
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
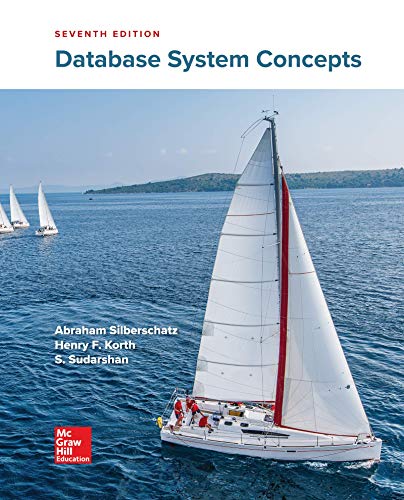
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
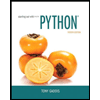
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
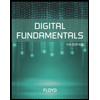
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
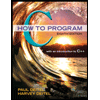
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
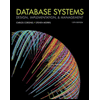
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
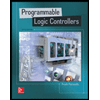
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education