You are given a template to help you follow the required structure. There are 10 files for the base and derived classes with specification and implementation files You are also given a driver MagicalCreaturesMain.cpp that you will complete. So 11 files in all. MagicalCreatureHeader.h // given to you MagicalCreature.cpp // given to you ElfHeader.h // given to you Elf.cpp // you implement methods DragonHeader.h // given to you Dragon.cpp // you implement methods GoblinHeader.h // given to you Goblin.cpp // you implement methods GenieHeader.h // given to you Genie.cpp // you implement methods Specifications: Here are five UML Diagrams: One for the Base class and the others for the derived classes. Use these along with the given template to complete the design of the classes and create the application. The template contains comments and notation as to where you need to fill in the code; Magical Creature (Base Class) -string name // name of the creature -string type // type of the creature -string color // color of the creature -int age // age of the creature + MagicalCreature() //default constructor that sets name, type,color to “null and age to 0 +MagicalCreature(string name, string type, string color, int age) // regular constructor +getName(): string // returns the name – can be any fun name +getType: string // returns type (Can be “Dragon”, “Genie” , ”Goblin”, “Elf”) +getAge: int // returns age. This should be a positive number +setAge(int age): void // can grow older or younger magically! +getColor() : string // returns any color +setColor(string color): void // can change color magically! +talk():string // returns creature speak +liveIn():string // return its natural habitat +toString(): String // return the object description as “Name: “ name “Age:” age “Type:” type “Color:” balance Dragon derived from Magical Creatures -bool hasSpikes // color of the dragon -int size // weight in pounds of the dragon + Dragon() //default constructor that sets name, type,color to “null and age to 0 + Dragon(string name,string color,string type, int age, int size, bool hasSpike); (string name, string type, string color, int age) // regular constructor getSize(): int // get size changeSize(int newSize): void // grows big or small! getHasSpike(): bool //set to true setHasSpike(bool hasSpike) // set to true toString():string // returns “I am a Dragon, I breathe fire!” Elf derived from Magical Creatures -int size // weight in pounds of the dragon + Elf() //default constructor that sets name, type,color to “null and age to 0 + Elf(string name,string color,string type, int age); (string name, string type, string color, int age) // regular constructor +toString():string // returns “I am an Elf..I can spell” Goblin derived from Magical Creatures + Goblin () //default constructor that sets name, type,color to “null and age to 0 + Goblin(string name, string color, string type, int age) // regular +talk():string // returns “I speak Gibberish” Genie derived from Magical Creatures -bool hasWand // set to true -int size // weight in pounds of the genie + Genie() //default constructor that sets name, type,color to “null and age to 0 +Genie(string name, string color, string type, int age, int size, bool +liveIn():string// return “I live in a bottle” getSize(): int; changeSize(int newSize): int getHasWand(): bool setHasWand(bool hasWand):void
You are given a template to help you follow the required structure. There are 10 files for the base and derived classes with specification and implementation files
You are also given a driver MagicalCreaturesMain.cpp that you will complete. So 11 files in all.
MagicalCreatureHeader.h // given to you
MagicalCreature.cpp // given to you
ElfHeader.h // given to you
Elf.cpp // you implement methods
DragonHeader.h // given to you
Dragon.cpp // you implement methods
GoblinHeader.h // given to you
Goblin.cpp // you implement methods
GenieHeader.h // given to you
Genie.cpp // you implement methods
Specifications:
Here are five UML Diagrams: One for the Base class and the others for the derived classes. Use these along with the given template to complete the design of the classes and create the application.
The template contains comments and notation as to where you need to fill in the code;
Magical Creature (Base Class) |
-string name // name of the creature -string type // type of the creature -string color // color of the creature -int age // age of the creature
|
+ MagicalCreature() //default constructor that sets name, type,color to “null and age to 0 +MagicalCreature(string name, string type, string color, int age) // regular constructor +getName(): string // returns the name – can be any fun name +getType: string // returns type (Can be “Dragon”, “Genie” , ”Goblin”, “Elf”) +getAge: int // returns age. This should be a positive number +setAge(int age): void // can grow older or younger magically! +getColor() : string // returns any color +setColor(string color): void // can change color magically! +talk():string // returns creature speak +liveIn():string // return its natural habitat +toString(): String // return the object description as “Name: “ name “Age:” age “Type:” type “Color:” balance |
Dragon derived from Magical Creatures |
-bool hasSpikes // color of the dragon -int size // weight in pounds of the dragon
|
+ Dragon() //default constructor that sets name, type,color to “null and age to 0 + Dragon(string name,string color,string type, int age, int size, bool hasSpike); (string name, string type, string color, int age) // regular constructor getSize(): int // get size changeSize(int newSize): void // grows big or small! getHasSpike(): bool //set to true setHasSpike(bool hasSpike) // set to true toString():string // returns “I am a Dragon, I breathe fire!”
|
Elf derived from Magical Creatures |
-int size // weight in pounds of the dragon
|
+ Elf() //default constructor that sets name, type,color to “null and age to 0 + Elf(string name,string color,string type, int age); (string name, string type, string color, int age) // regular constructor +toString():string // returns “I am an Elf..I can spell”
|
Goblin derived from Magical Creatures |
|
+ Goblin () //default constructor that sets name, type,color to “null and age to 0 + Goblin(string name, string color, string type, int age) // regular +talk():string // returns “I speak Gibberish” |
Genie derived from Magical Creatures |
-bool hasWand // set to true -int size // weight in pounds of the genie
|
+ Genie() //default constructor that sets name, type,color to “null and age to 0 +Genie(string name, string color, string type, int age, int size, bool +liveIn():string// return “I live in a bottle” getSize(): int; changeSize(int newSize): int getHasWand(): bool setHasWand(bool hasWand):void |


Step by step
Solved in 2 steps with 1 images

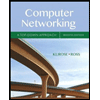
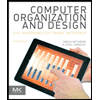
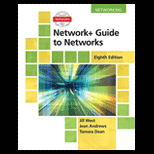
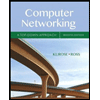
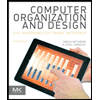
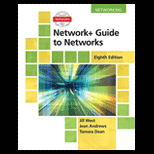
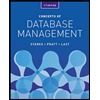
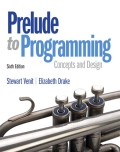
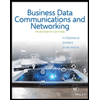