Your company, A to Z Logistics, is in charge of packing shipping containers. Every container has two large compartments. The items being shipped go evenly into the two compartments. Each item is encoded as a single character, a-z (lowercase) or A-Z (uppercase). Your job is to write a program to calculate the shipping fees based on the items that go into the containers. Specification Your program will be provided, as input, one or more strings. Each container string contains only letters and it represents what's to be put into a container. A string that starts with a dot (it will be the only character in the string) will mark the end of input. Here is a sample input, consisting of four container strings and the input-terminating string: bmaBbZjRvs PBZRiwlHcmbMRMUSTaCF xpIJDqWoMnisOSFY KLUSgJFedAkFfScuVRYxekDgsc Because of limitations on container size, no string will be longer than 100 characters. Each letter corresponds to an item whose weight is determined by the table here: Letter a lb C ... Z A B Z Weight 0.1 kg 0.2 kg 0.3 kg 2.6 kg 2.7 kg 2.8 kg 5.2 kg In short, each successive letter represents an increase in weight of 0.1 kg, starting with 0.1 kg for "a" up to 5.2 kg for "Z". Since the items in each container are split among the two compartments, the first half of the string represents the items in the one compartment and the second half are the items in the other compartment. To calculate the billable weight, sum up the weights of the items in each compartment. Only the greater of the two weights will be used to calculate the shipping fee. The shipping fee is billed at $2.75 per kilogram. When input has ended (signified by the single dot on a line by itself), the program displays the total shipping fee, displayed as a dollar amount with two decimal places
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
Need help writing this in C language



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

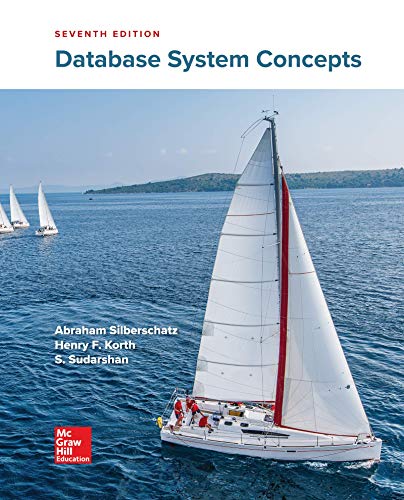
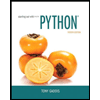
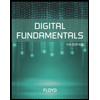
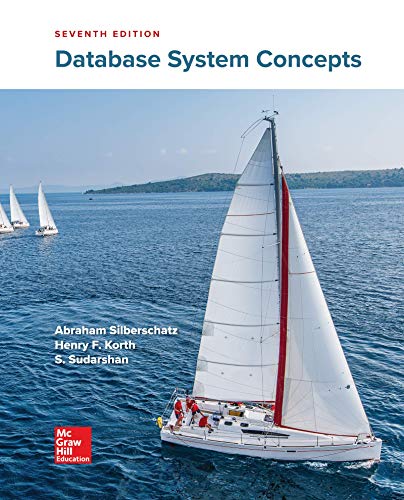
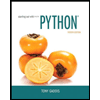
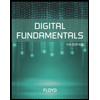
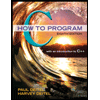
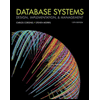
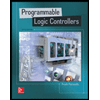