CIS 2275 C++ Programming Part I Program 6 Trivia Game In this program, create a simple trivia game for two players. It will work like this: Starting with player 1, each player gets a turn at answering five trivia questions. There are a total of 10 questions. When a question is displayed, four possible answers are also displayed. Only one of the answers is correct, and if the player selects the correct answer, they earn a point. After the answers have been selected for all of the questions, the program displays the number of points earned by each player and declare the player with the highest number of points to be the winner. The players will have the option of playing another round. The flow of the program will be: 1. Show the Header information. 2. Create an array of Question structs. 3. Read the questions from the text file. 4. If the questions were properly read, start a do-while loop. 5. Declare and initialize: a. playerOnePoints and playerTwoPoints b. questionNumber 6. start a while loop - while questionNumber is less than 10 7. Identify player One is being asked the question with a cout 8. call the function PlayARound(the Question struct variable, the questionNumber, the playerOnePoints) 9. Identify player Two is being asked the question with a cout. 10. call the function PlayARound(the Question struct variable, the questionNumber, playerTwoPoints) 11. When the control exits out of the while loop, call ShowFinalScores(playerOnePoints, playerTwoPoints); 12. Ask the player if they want to play again. If yes, loop up to the top of the do-while loop. If no, drop out and say good-bye. 13. return 0; Functions.h file contains: Constants for filename, number of questions (10), and number of answers (4) The Question struct declaration: struct Question { string question; string answers[NUM_ANSWERS]; int correctIndex; //the correct index for the answer }; Function Prototypes: bool InitQuestions(Question questions[]); void DisplayQuestion(Question questions[], int index); bool CheckAnswer(Question questions[], int questionNum, int playerAnswer); void PlayARound(Question quest[], int &questionNum, int &playerPoints); void ShowFinalScores(int playerOnePoints, int playerTwoPoints); InitQuestions will; a. Read a text file which contains the questions and answers and the correct index. The filename may be contained in the constant in the .h file. b. When the file is opened, test to make sure it is valid. If not, return false. c. If true, read the text into the appropriate locations in the struct array element. d. return true. PlayARound will: a. Call DisplayQuestion. b. Ask the user to enter the answer number. c. Read in the user answer, make sure the answer is in range. If the user answer is not in range let them know that their answer is not in range and ask until their answer is in range. d. Call CheckAnswer and assign the return into a bool. e. Check the bool and if it is true, increment the player’s points. f. Increment the questionNumber by 1. DisplayQuestion will: a. cout the question string. b. cout a heading that states answers. c. cout the 4 answer strings. CheckAnswer will: a. check if the index selected by the plays matches the index that is correct. b. cout a message of success or failure and if the player is incorrect, give the correct answer. c. Return true if the player was correct and false otherwise. ShowFinalScores will, using nice formatting: a. cout the points for each player and announces who won or if they tied. b. cout the number of games won by each player
CIS 2275 C++ Programming Part I
Program 6 Trivia Game
In this program, create a simple trivia game for two players. It will work like this:
Starting with player 1, each player gets a turn at answering five trivia questions. There are a total of 10 questions. When a question is displayed, four possible answers are also displayed. Only one of the answers is correct, and if the player selects the correct answer, they earn a point.
After the answers have been selected for all of the questions, the program displays the number of points earned by each player and declare the player with the highest number of points to be the winner. The players will have the option of playing another round.
The flow of the program will be:
1. Show the Header information.
2. Create an array of Question structs.
3. Read the questions from the text file.
4. If the questions were properly read, start a do-while loop.
5. Declare and initialize:
a. playerOnePoints and playerTwoPoints
b. questionNumber
6. start a while loop - while questionNumber is less than 10
7. Identify player One is being asked the question with a cout
8. call the function PlayARound(the Question struct variable, the questionNumber, the playerOnePoints)
9. Identify player Two is being asked the question with a cout.
10. call the function PlayARound(the Question struct variable, the questionNumber, playerTwoPoints)
11. When the control exits out of the while loop, call ShowFinalScores(playerOnePoints, playerTwoPoints);
12. Ask the player if they want to play again. If yes, loop up to the top of the do-while loop. If no, drop out and say good-bye.
13. return 0;
Functions.h file contains:
Constants for filename, number of questions (10), and number of answers (4)
The Question struct declaration:
struct Question
{
string question;
string answers[NUM_ANSWERS];
int correctIndex; //the correct index for the answer
};
Function Prototypes:
bool InitQuestions(Question questions[]);
void DisplayQuestion(Question questions[], int index);
bool CheckAnswer(Question questions[], int questionNum, int playerAnswer);
void PlayARound(Question quest[], int &questionNum, int &playerPoints);
void ShowFinalScores(int playerOnePoints, int playerTwoPoints);
InitQuestions will;
a. Read a text file which contains the questions and answers and the correct index. The filename may be contained in the constant in the .h file.
b. When the file is opened, test to make sure it is valid. If not, return false.
c. If true, read the text into the appropriate locations in the struct array element.
d. return true.
PlayARound will:
a. Call DisplayQuestion.
b. Ask the user to enter the answer number.
c. Read in the user answer, make sure the answer is in range. If the user answer is not in range let them know that their answer is not in range and ask until their answer is in range.
d. Call CheckAnswer and assign the return into a bool.
e. Check the bool and if it is true, increment the player’s points.
f. Increment the questionNumber by 1.
DisplayQuestion will:
a. cout the question string.
b. cout a heading that states answers.
c. cout the 4 answer strings.
CheckAnswer will:
a. check if the index selected by the plays matches the index that is correct.
b. cout a message of success or failure and if the player is incorrect, give the correct answer.
c. Return true if the player was correct and false otherwise.
ShowFinalScores will, using nice formatting:
a. cout the points for each player and announces who won or if they tied.
b. cout the number of games won by each player

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 9 images

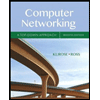
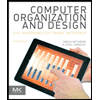
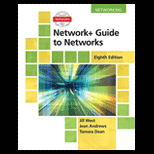
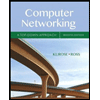
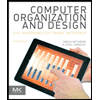
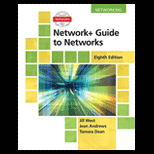
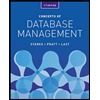
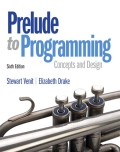
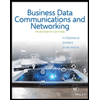