Draw the UML class diagrams for the following classes: An abstract Java class called Person that has the following attributes: a String called idNumber a Date called dateOfBirth representing the date of birth. a String for name A class called VaccineRecord with the following attributes: an enum called type of VaccineType a Date called date a String called batchCode A class called Patient that extends the Person class and has the following attributes and behaviours: an ArrayList that contains VaccineRecord objects called vaccinationRecord a public method called vaccinate that takes a VaccineRecord with the following signature: public void vaccinate(VaccineRecord vaccineRecord); a public boolean method called isVaccinated that returns whether or not the Patient has had a vaccine. A MedicalPractitioner class that extends the Person class and has the following attributes and behaviours: a String called licenseCode a public method called vaccinatePatient that takes as a parameter a Patient object, a VaccineType, and a String batchCode The class Person has the following implementations in addition to those specified above: the dateOfBirth attribute is of type java.util.Date public getter methods for the attributes a public constructor Person with the following method signature: public Person(String idNumber, String name, Date dateOfBirth) declare an abstract compareTo method in Person with the following signature: public int compareTo(Person p); The class called VaccineRecord has the following extra details: the enum called VaccineType has the following possible values: JJ, AZ, MD, PF the public constructor for the VaccineRecord has the following signature: public VaccineRecord(VaccineType type, String batchCode); the date attribute is of type java.util.Date The class called Patient that extends the Person class has the following additional attributes and behaviours: the public method called vaccinate takes a VaccineRecord and adds it to the vaccinationRecord list a constructor with the following method signature: public Patient(String idNumber, String name, Date dateOfBirth); The class called MedicalPractitioner has the following additional attributes and behaviours: a public constructor with the following method signature: public MedicalPractitioner(String idNumber, String name, Date dateOfBirth, String licenseCode); the vaccinate method creates a new VaccineRecord using the VaccineType and batchCode and calls the vaccinate method on the Patient. The method signature is as follows: public void vaccinate(VaccineRecord.VaccineType vaccineType, String batchCode, Patient patient); Sample Input/Output The following demo code produces the sample output: import java.util.Date; publicclassAssignmentDemo { publicstaticvoidmain(String[] args) { MedicalPractitionernurseJoy=newMedicalPractitioner("6405013145087", "Nurse Joy", newDate(-178934400000L), "p0k3m0n-k4nt0"); PatientashKetchum=newPatient("9906014269088", "Ash Ketchum", newDate(928195200000L)); System.out.printf("Name: %s\nRSA ID:%s\nDOB:%s\nVaccinated?: %b\n", ashKetchum.getName(), ashKetchum.getIdNumber(), ashKetchum.getDateOfBirth(), ashKetchum.isVaccinated()); nurseJoy.vaccinate(VaccineRecord.VaccineType.JJ, "batch-x0j2", ashKetchum); System.out.println("----------------------------"); System.out.printf("Name: %s\nRSA ID:%s\nDOB:%s\nVaccinated?: %b\n", ashKetchum.getName(), ashKetchum.getIdNumber(), ashKetchum.getDateOfBirth(), ashKetchum.isVaccinated()); } } Name: Ash Ketchum RSA ID:9906014269088 DOB:Tue Jun 01 02:00:00 SAST 1999 Vaccinated?: false ---------------------------- Name: Ash Ketchum RSA ID:9906014269088 DOB:Tue Jun 01 02:00:00 SAST 1999 Vaccinated?: true
Draw the UML class diagrams for the following classes: An abstract Java class called Person that has the following attributes: a String called idNumber a Date called dateOfBirth representing the date of birth. a String for name A class called VaccineRecord with the following attributes: an enum called type of VaccineType a Date called date a String called batchCode A class called Patient that extends the Person class and has the following attributes and behaviours: an ArrayList that contains VaccineRecord objects called vaccinationRecord a public method called vaccinate that takes a VaccineRecord with the following signature: public void vaccinate(VaccineRecord vaccineRecord); a public boolean method called isVaccinated that returns whether or not the Patient has had a vaccine. A MedicalPractitioner class that extends the Person class and has the following attributes and behaviours: a String called licenseCode a public method called vaccinatePatient that takes as a parameter a Patient object, a VaccineType, and a String batchCode The class Person has the following implementations in addition to those specified above: the dateOfBirth attribute is of type java.util.Date public getter methods for the attributes a public constructor Person with the following method signature: public Person(String idNumber, String name, Date dateOfBirth) declare an abstract compareTo method in Person with the following signature: public int compareTo(Person p); The class called VaccineRecord has the following extra details: the enum called VaccineType has the following possible values: JJ, AZ, MD, PF the public constructor for the VaccineRecord has the following signature: public VaccineRecord(VaccineType type, String batchCode); the date attribute is of type java.util.Date The class called Patient that extends the Person class has the following additional attributes and behaviours: the public method called vaccinate takes a VaccineRecord and adds it to the vaccinationRecord list a constructor with the following method signature: public Patient(String idNumber, String name, Date dateOfBirth); The class called MedicalPractitioner has the following additional attributes and behaviours: a public constructor with the following method signature: public MedicalPractitioner(String idNumber, String name, Date dateOfBirth, String licenseCode); the vaccinate method creates a new VaccineRecord using the VaccineType and batchCode and calls the vaccinate method on the Patient. The method signature is as follows: public void vaccinate(VaccineRecord.VaccineType vaccineType, String batchCode, Patient patient); Sample Input/Output The following demo code produces the sample output: import java.util.Date; publicclassAssignmentDemo { publicstaticvoidmain(String[] args) { MedicalPractitionernurseJoy=newMedicalPractitioner("6405013145087", "Nurse Joy", newDate(-178934400000L), "p0k3m0n-k4nt0"); PatientashKetchum=newPatient("9906014269088", "Ash Ketchum", newDate(928195200000L)); System.out.printf("Name: %s\nRSA ID:%s\nDOB:%s\nVaccinated?: %b\n", ashKetchum.getName(), ashKetchum.getIdNumber(), ashKetchum.getDateOfBirth(), ashKetchum.isVaccinated()); nurseJoy.vaccinate(VaccineRecord.VaccineType.JJ, "batch-x0j2", ashKetchum); System.out.println("----------------------------"); System.out.printf("Name: %s\nRSA ID:%s\nDOB:%s\nVaccinated?: %b\n", ashKetchum.getName(), ashKetchum.getIdNumber(), ashKetchum.getDateOfBirth(), ashKetchum.isVaccinated()); } } Name: Ash Ketchum RSA ID:9906014269088 DOB:Tue Jun 01 02:00:00 SAST 1999 Vaccinated?: false ---------------------------- Name: Ash Ketchum RSA ID:9906014269088 DOB:Tue Jun 01 02:00:00 SAST 1999 Vaccinated?: true
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Draw the UML class diagrams for the following classes:
- An abstract Java class called Person that has the following attributes:
- a String called idNumber
- a Date called dateOfBirth representing the date of birth.
- a String for name
- A class called VaccineRecord with the following attributes:
- an enum called type of VaccineType
- a Date called date
- a String called batchCode
- A class called Patient that extends the Person class and has the following attributes and behaviours:
- an ArrayList that contains VaccineRecord objects called vaccinationRecord
- a public method called vaccinate that takes a VaccineRecord with the following signature:
public void vaccinate(VaccineRecord vaccineRecord);- a public boolean method called isVaccinated that returns whether or not the Patient has had a vaccine.
- A MedicalPractitioner class that extends the Person class and has the following attributes and behaviours:
- a String called licenseCode
- a public method called vaccinatePatient that takes as a parameter a Patient object, a VaccineType, and a String batchCode
- The class Person has the following implementations in addition to those specified above:
- the dateOfBirth attribute is of type java.util.Date
- public getter methods for the attributes
- a public constructor Person with the following method signature:
public Person(String idNumber, String name, Date dateOfBirth)- declare an abstract compareTo method in Person with the following signature:
public int compareTo(Person p); - The class called VaccineRecord has the following extra details:
- the enum called VaccineType has the following possible values:
- the public constructor for the VaccineRecord has the following signature:
- the date attribute is of type java.util.Date
- The class called Patient that extends the Person class has the following additional attributes and behaviours:
- the public method called vaccinate takes a VaccineRecord and adds it to the vaccinationRecord list
- a constructor with the following method signature:
public Patient(String idNumber, String name, Date dateOfBirth); - The class called MedicalPractitioner has the following additional attributes and behaviours:
- a public constructor with the following method signature:
public MedicalPractitioner(String idNumber, String name, Date dateOfBirth, String licenseCode);- the vaccinate method creates a new VaccineRecord using the VaccineType and batchCode and calls the vaccinate method on the Patient. The method signature is as follows:
public void vaccinate(VaccineRecord.VaccineType vaccineType, String batchCode, Patient patient);
Sample Input/Output
The following demo code produces the sample output:
import java.util.Date; publicclassAssignmentDemo { publicstaticvoidmain(String[] args) { MedicalPractitionernurseJoy=newMedicalPractitioner("6405013145087", "Nurse Joy", newDate(-178934400000L), "p0k3m0n-k4nt0"); PatientashKetchum=newPatient("9906014269088", "Ash Ketchum", newDate(928195200000L)); System.out.printf("Name: %s\nRSA ID:%s\nDOB:%s\nVaccinated?: %b\n", ashKetchum.getName(), ashKetchum.getIdNumber(), ashKetchum.getDateOfBirth(), ashKetchum.isVaccinated()); nurseJoy.vaccinate(VaccineRecord.VaccineType.JJ, "batch-x0j2", ashKetchum); System.out.println("----------------------------"); System.out.printf("Name: %s\nRSA ID:%s\nDOB:%s\nVaccinated?: %b\n", ashKetchum.getName(), ashKetchum.getIdNumber(), ashKetchum.getDateOfBirth(), ashKetchum.isVaccinated()); } }
Name: Ash Ketchum RSA ID:9906014269088 DOB:Tue Jun 01 02:00:00 SAST 1999 Vaccinated?: false ---------------------------- Name: Ash Ketchum RSA ID:9906014269088 DOB:Tue Jun 01 02:00:00 SAST 1999 Vaccinated?: true
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
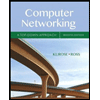
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
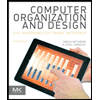
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
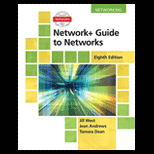
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
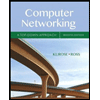
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
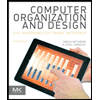
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
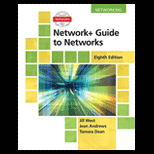
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
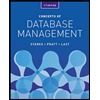
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
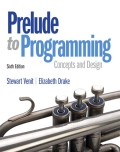
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
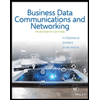
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY