ee Snip for detail of question. Template.py: import turtle as t class CenteredPolygon: def __init__(self, n, size, center=(0, 0), pen=None, **kwargs): self.numsides = n self.size = size self.center = center self.corners = [] self.pen = pen if pen is not None else self.init_pen(**kwargs) self.draw() def init_pen(self, **kwargs): pen = t.Turtle() for key, value in kwargs.items(): try: f = getattr(pen, key) f(value) except Exception as e: print(e) return pen def set_corners(self) -> list: p = self.pen p.pu() p.goto(self.center) for i in range(self.numsides): p.forward(self.size/2) self.corners.append(p.pos()) p.back(self.size/2) p.left(360/self.numsides) p.goto(self.center) return self.corners def draw(self): if not self.corners: self.set_corners() p = self.pen # p.clear() p.pu() p.goto(self.corners[0]) p.pd() p.begin_fill() for i in self.corners: p.goto(i) p.goto(self.corners[0]) p.end_fill() p.pu() p.goto(self.center) p.ht() t.update() class MyPolygon(CenteredPolygon): def rotate_by(self, theta, degrees=False): ... if __name__ == '__main__': # DO NOT EDIT t.tracer(100) import random, time from random import randint as r random.seed(3) n = 3 col = lambda: "#"+"".join(f"{r(0, 255):02x}" for _ in range(3)) polys = [] size = 500 for i in range(6): polys.append(MyPolygon(n, size, (0, 0), color=col())) size -= 50 while True: for a in range(0, 180, 90): for p in polys: p.rotate_by(a, degrees=True) a *= -1 t.update() time.sleep(0.1)
ee Snip for detail of question. Template.py: import turtle as t class CenteredPolygon: def __init__(self, n, size, center=(0, 0), pen=None, **kwargs): self.numsides = n self.size = size self.center = center self.corners = [] self.pen = pen if pen is not None else self.init_pen(**kwargs) self.draw() def init_pen(self, **kwargs): pen = t.Turtle() for key, value in kwargs.items(): try: f = getattr(pen, key) f(value) except Exception as e: print(e) return pen def set_corners(self) -> list: p = self.pen p.pu() p.goto(self.center) for i in range(self.numsides): p.forward(self.size/2) self.corners.append(p.pos()) p.back(self.size/2) p.left(360/self.numsides) p.goto(self.center) return self.corners def draw(self): if not self.corners: self.set_corners() p = self.pen # p.clear() p.pu() p.goto(self.corners[0]) p.pd() p.begin_fill() for i in self.corners: p.goto(i) p.goto(self.corners[0]) p.end_fill() p.pu() p.goto(self.center) p.ht() t.update() class MyPolygon(CenteredPolygon): def rotate_by(self, theta, degrees=False): ... if __name__ == '__main__': # DO NOT EDIT t.tracer(100) import random, time from random import randint as r random.seed(3) n = 3 col = lambda: "#"+"".join(f"{r(0, 255):02x}" for _ in range(3)) polys = [] size = 500 for i in range(6): polys.append(MyPolygon(n, size, (0, 0), color=col())) size -= 50 while True: for a in range(0, 180, 90): for p in polys: p.rotate_by(a, degrees=True) a *= -1 t.update() time.sleep(0.1)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
See Snip for detail of question.
Template.py:
import turtle as t
class CenteredPolygon:
def __init__(self, n, size, center=(0, 0), pen=None, **kwargs):
self.numsides = n
self.size = size
self.center = center
self.corners = []
self.pen = pen if pen is not None else self.init_pen(**kwargs)
self.draw()
def init_pen(self, **kwargs):
pen = t.Turtle()
for key, value in kwargs.items():
try:
f = getattr(pen, key)
f(value)
except Exception as e:
print(e)
return pen
def set_corners(self) -> list:
p = self.pen
p.pu()
p.goto(self.center)
for i in range(self.numsides):
p.forward(self.size/2)
self.corners.append(p.pos())
p.back(self.size/2)
p.left(360/self.numsides)
p.goto(self.center)
return self.corners
def draw(self):
if not self.corners:
self.set_corners()
p = self.pen
# p.clear()
p.pu()
p.goto(self.corners[0])
p.pd()
p.begin_fill()
for i in self.corners:
p.goto(i)
p.goto(self.corners[0])
p.end_fill()
p.pu()
p.goto(self.center)
p.ht()
t.update()
class MyPolygon(CenteredPolygon):
def rotate_by(self, theta, degrees=False):
...
if __name__ == '__main__':
# DO NOT EDIT
t.tracer(100)
import random, time
from random import randint as r
random.seed(3)
n = 3
col = lambda: "#"+"".join(f"{r(0, 255):02x}" for _ in range(3))
polys = []
size = 500
for i in range(6):
polys.append(MyPolygon(n, size, (0, 0), color=col()))
size -= 50
while True:
for a in range(0, 180, 90):
for p in polys:
p.rotate_by(a, degrees=True)
a *= -1
t.update()
time.sleep(0.1)

Transcribed Image Text:Using the given template.py script define the class MyPolygon as prescribed in the diagram below. The rotate method must change the orientation of the drawn shape by a specified angle (given by default in radians).
NB: Do not edit the code under main
<block
CenteredPolygon
<blocka
MyPolygon
operations
rotate_by( theta, degree False)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
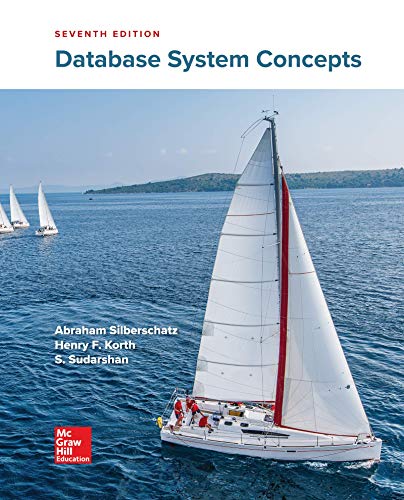
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
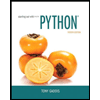
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
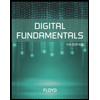
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
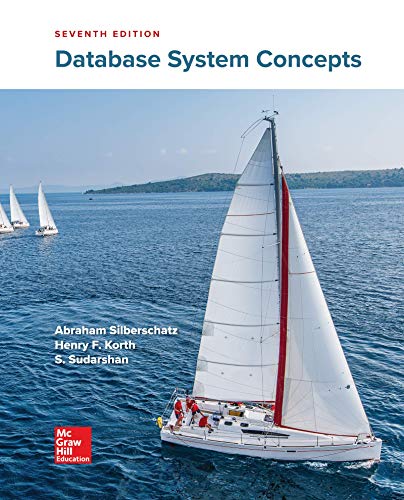
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
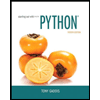
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
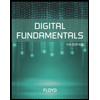
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
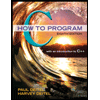
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
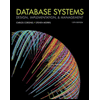
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
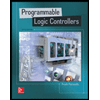
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education