In this laboratory work, you are expected to write a c# console application to make some operations about the customers. Operations and required featurest that you need to cover in your program are following: Add: This function adds a new person to json file. You need to get inputs from user and adds the new one into json file. Display: This function displays all people in your json file. • Search: This function let you search with the user id. • Create Password: This function will create a random password for the user and user may choose the length of the password. Password may include numbers and
In this laboratory work, you are expected to write a c# console application to make some operations about the customers. Operations and required featurest that you need to cover in your program are following: Add: This function adds a new person to json file. You need to get inputs from user and adds the new one into json file. Display: This function displays all people in your json file. • Search: This function let you search with the user id. • Create Password: This function will create a random password for the user and user may choose the length of the password. Password may include numbers and
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:In this laboratory work, you are expected to write a c# console application to make some
operations about the customers. Operations and required featurest that you need to cover in
your program are following:
• Add: This function adds a new person to json file. You need to get inputs from user
and adds the new one into json file.
• Display: This function displays all people in your json file.
• Search: This function let you search with the user id.
• Create Password: This function will create a random password for the user and user
may choose the length of the password. Password may include numbers and
characters.
• Exceptions: You need to handle exceptions as well. You should at least handle 3 of
exceptional cases. For the case that file can not be found, It is mandatory to catch the
exception.
Sample json string:
"FirstName": "Kaan",
"LastName": "OZAYDIN",
"EmployeeId": 101,
"Email": "",
"Password":
"Address": (
"ZipCode": "06460",
"State": "Ankara",
"Country": "Turkey"
"FirstName": "Erkan",
"LastName": "YILDIZ",
"EmployeeId": 102,
"Email": "".
"Password":
"Address": {
"ZipCode": "06460",
"State": "Ankara",
"Country": "Turkey"
Above you may see the structure of json file you need to use.

Transcribed Image Text:In your main class, you first need to read the json file. Then, you should create a list of
objects. You are expected to deserialize your json to list. After that you should create another
json file that has the updated string. You may see the flow in the below:
| Seç Microsoft Visual Studio Hata Ayıklama Konsolu
Current File:
101
Kaan
OZAYDIN
Turkey
Ankara
06460
102
Erkan
YILDIZ
Turkey
Ankara
06460
please choose an operation: add, display, search, ereatePassword, exit
add
First Name:
Emre
Last Name:
Gokmen
Idi
103
Email:
emregkmnegmail.com
Country
Тurkey
Statei
Ankara
Zipcode
06470
Figure 1: Sample Output.
paease cnoose an operataoni aaa, aaspaay, searen, createrasswora, exat
display
101
Kaan
OZAYDIN
Turkey
Ankara
06460
102
Erkan
YILDIZ
Turkey
Ankara
06460
103
Eere
Goknen
Turkey
Ankara
06470
please choose an operation: add, display, search, createPassword, exit
search
Please anter id of the customar
103
103
Emre
Goknen
Turkey
Ankara
06470
please choose an operationi add, display, search, creataPassword, exit
createPassvord
Please enter id of the customer
103
Enter the length of passuord
please choose an operation: add, display, search, createPassword, exit
Ci\Users\kaan\source\repos\jsonLab\jsonLab\bin\Debug\netcoreapp3.1\jsonLab.exe (22348 islemi), e koduyla çakas yapta.
Bu pencereyi kapatmak 1çin bir tusa basan...
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
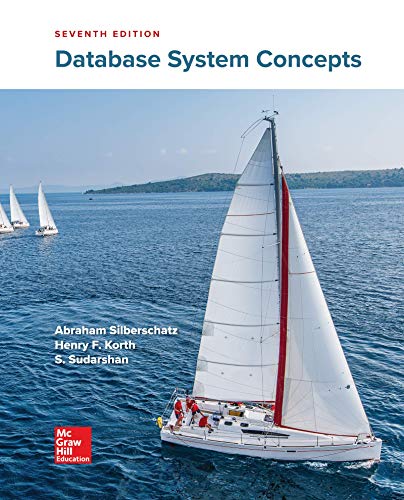
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
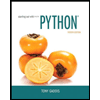
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
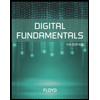
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
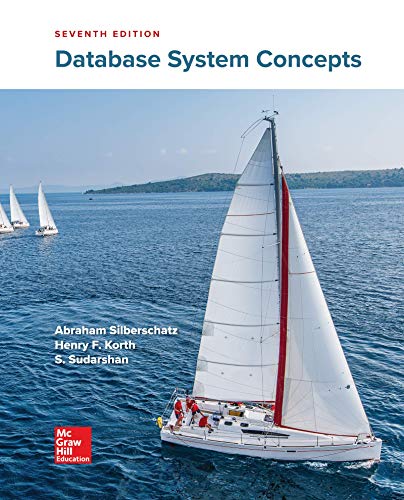
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
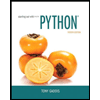
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
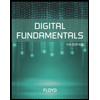
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
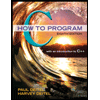
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
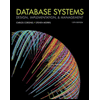
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
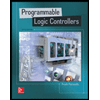
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education