Java programming Help Need help with question: P 8.61 P 8.61: Implement the binary tree ADT using the array-based representation described in Section 8.3.2.
Java programming Help Need help with question: P 8.61 P 8.61: Implement the binary tree ADT using the array-based representation described in Section 8.3.2.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Java
Need help with question: P 8.61
P 8.61: Implement the binary tree ADT using the array-based representation described in Section 8.3.2.

Transcribed Image Text:8.3.2 Array-Based Representation of a Binary Tree
An alternative representation of a binary tree T is based on a way of numbering the
positions of T. For every position p of T, let f(p) be the integer defined as follows.
• If p is the root of T, then f(p) = 0.
• If p is the left child of position q, then ƒ(p) = 2ƒ(q)+1.
• If p is the right child of position q, then ƒ(p) = 2ƒ(q) +2.
The numbering function f is known as a level numbering of the positions in a
binary tree T, for it numbers the positions on each level of T in increasing order
from left to right. (See Figure 8.10.) Note well that the level numbering is based
on potential positions within a tree, not the actual shape of a specific tree, so they
are not necessarily consecutive. For example, in Figure 8.10(b), there are no nodes
with level numbering 13 or 14, because the node with level numbering 6 has no
children.
(a)
(b)
15
3
7
+
16
1
3
*
8
3
4
19
9
10 11
9
20
5
10
5
12 13
3
*
25
7
6
12
14
26
4
+
6
6
Figure 8.10: Binary tree level numbering: (a) general scheme; (b) an example.
![The level numbering function f suggests a representation of a binary tree T by
means of an array-based structure A, with the element at position p of T stored at
index f(p) of the array. We show an example of an array-based representation of a
binary tree in Figure 8.11.
7
3
3
8
/ * ++4
0 1 2
3
4
1
*
4
4
11.
9
5
2
+
12
5
6
2
2 3 1
95
5 6 7 8 9 10 11 12 13 14
Figure 8.11: Representation of a binary tree by means of an array.
One advantage of an array-based representation of a binary tree is that a posi-
tion p can be represented by the single integer f(p), and that position-based meth-
ods such as root, parent, left, and right can be implemented using simple arithmetic
operations on the number f(p). Based on our formula for the level numbering, the
left child of p has index 2ƒ(p) +1, the right child of p has index 2ƒ(p) +2, and
the parent of p has index [(ƒ(p) — 1)/2]. We leave the details of a complete array-
based implementation as Exercise R-8.16.
The space usage of an array-based representation depends greatly on the shape
of the tree. Let n be the number of nodes of T, and let f be the maximum value
of f(p) over all the nodes of T. The array A requires length N = 1 + fm, since
elements range from A[0] to A[fM]. Note that A may have a number of empty cells
that do not refer to existing positions of T. In fact, in the worst case, N = 2¹ - 1,
the justification of which is left as an exercise (R-8.14). In Section 9.3, we will
see a class of binary trees, called "heaps" for which N = n. Thus, in spite of the
worst-case space usage, there are applications for which the array representation
of a binary tree is space efficient. Still, for general binary trees, the exponential
worst-case space requirement of this representation is prohibitive.
Another drawback of an array representation is that many update operations for
trees cannot be efficiently supported. For example, removing a node and promoting
its child takes O(n) time because it is not just the child that moves locations within
the array, but all descendants of that child.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe8f98c03-2dea-45fe-9e7d-c001811b98bd%2F9e2eb371-972a-4fd3-9ae7-e77737d4d301%2Flqlenni_processed.png&w=3840&q=75)
Transcribed Image Text:The level numbering function f suggests a representation of a binary tree T by
means of an array-based structure A, with the element at position p of T stored at
index f(p) of the array. We show an example of an array-based representation of a
binary tree in Figure 8.11.
7
3
3
8
/ * ++4
0 1 2
3
4
1
*
4
4
11.
9
5
2
+
12
5
6
2
2 3 1
95
5 6 7 8 9 10 11 12 13 14
Figure 8.11: Representation of a binary tree by means of an array.
One advantage of an array-based representation of a binary tree is that a posi-
tion p can be represented by the single integer f(p), and that position-based meth-
ods such as root, parent, left, and right can be implemented using simple arithmetic
operations on the number f(p). Based on our formula for the level numbering, the
left child of p has index 2ƒ(p) +1, the right child of p has index 2ƒ(p) +2, and
the parent of p has index [(ƒ(p) — 1)/2]. We leave the details of a complete array-
based implementation as Exercise R-8.16.
The space usage of an array-based representation depends greatly on the shape
of the tree. Let n be the number of nodes of T, and let f be the maximum value
of f(p) over all the nodes of T. The array A requires length N = 1 + fm, since
elements range from A[0] to A[fM]. Note that A may have a number of empty cells
that do not refer to existing positions of T. In fact, in the worst case, N = 2¹ - 1,
the justification of which is left as an exercise (R-8.14). In Section 9.3, we will
see a class of binary trees, called "heaps" for which N = n. Thus, in spite of the
worst-case space usage, there are applications for which the array representation
of a binary tree is space efficient. Still, for general binary trees, the exponential
worst-case space requirement of this representation is prohibitive.
Another drawback of an array representation is that many update operations for
trees cannot be efficiently supported. For example, removing a node and promoting
its child takes O(n) time because it is not just the child that moves locations within
the array, but all descendants of that child.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
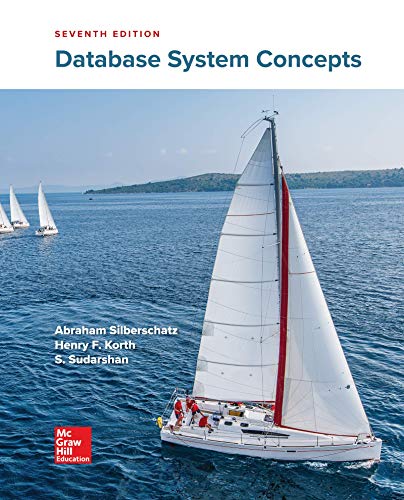
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
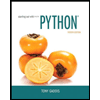
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
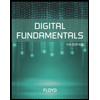
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
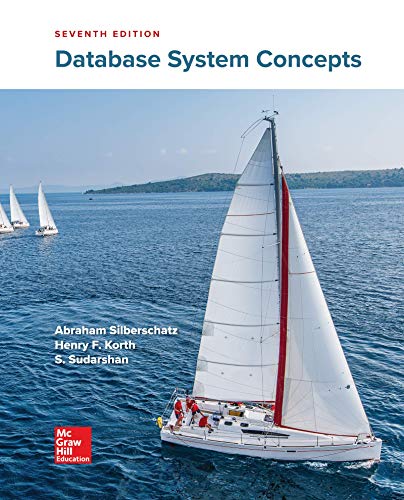
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
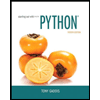
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
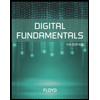
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
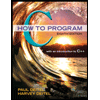
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
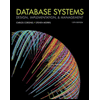
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
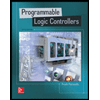
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education