Modify the program 11-10 from pages 753-755 as follows: 1. To Length class files (Length.h and Length.cpp) add the operator overload for stream insertion (<<) and stream extraction (>>) from page 759. You should not use the modified version of class Length from page 759 because it contains more operator overloads. Instead of typing you can use copy/paste from Length1.h and Length1.cpp respectively. 2. In main() replace the lines 11-16 with appropriate usage of stream extraction overload (>>) to change "first" and "second" objects with values inputted by the end user. 3. In main(), replace the output on lines 21,22 and 25,26 with appropriate usage of stream insertion (<<) operator. The resulting output should be identical with the unmodified version of the program. 11-10: // This program demonstrates the Length class's overloaded // +, -, ==, and < operators. #include #include "Length.h" using namespace std; int main() { Length first(0), second(0), third(0); int f, i; cout << "Enter a distance in feet and inches: "; cin >> f >> i; first.setLength(f, i); cout << "Enter another distance in feet and inches: "; cin >> f >> i; second.setLength(f, i); // Test the + and - operators third = first + second; cout << "first + second = "; cout << third.getFeet() << " feet, "; cout << third.getInches() << " inches.\n"; third = first - second; cout << "first - second = "; cout << third.getFeet() << " feet, "; cout << third.getInches() << " inches.\n"; // Test the relational operators cout << "first == second = "; if (first == second) cout << "true"; else cout << "false"; cout << "\n"; cout << "first < second = "; if (first < second) cout << "true"; else cout << "false"; cout << "\n"; return 0; } Length.cpp: #include "Length.h" //************************************* // Overloaded operator + * //************************************* Length operator+(Length a, Length b) { return Length(a.len_inches + b.len_inches); } //************************************* // Overloaded operator - * //************************************* Length operator-(Length a, Length b) { return Length(a.len_inches - b.len_inches); } //************************************ // Overloaded operator == * //************************************ bool operator==(Length a, Length b) { return a.len_inches == b.len_inches; } //************************************ // Overloaded operator < * //************************************ bool operator<(Length a, Length b) { return a.len_inches < b.len_inches; } Length.h: #ifndef _LENGTH_H #define _LENGTH_H #include using namespace std; class Length { private: int len_inches; public: Length(int feet, int inches) { setLength(feet, inches); } Length(int inches){ len_inches = inches; } int getFeet() const { return len_inches / 12; } int getInches() const { return len_inches % 12; } void setLength(int feet, int inches) { len_inches = 12 *feet + inches; } friend Length operator+(Length a, Length b); friend Length operator-(Length a, Length b); friend bool operator< (Length a, Length b); friend bool operator== (Length a, Length b); }; #endif
Modify the program 11-10 from pages 753-755 as follows:
1. To Length class files (Length.h and Length.cpp) add the operator overload for stream insertion (<<) and stream extraction (>>) from page 759. You should not use the modified version of class Length from page 759 because it contains more operator overloads. Instead of typing you can use copy/paste from Length1.h and Length1.cpp respectively.
2. In main() replace the lines 11-16 with appropriate usage of stream extraction overload (>>) to change "first" and "second" objects with values inputted by the end user.
3. In main(), replace the output on lines 21,22 and 25,26 with appropriate usage of stream insertion (<<) operator.
The resulting output should be identical with the unmodified version of the program.
11-10:
// This program demonstrates the Length class's overloaded
// +, -, ==, and < operators.
#include <iostream>
#include "Length.h"
using namespace std;
int main()
{
Length first(0), second(0), third(0);
int f, i;
cout << "Enter a distance in feet and inches: ";
cin >> f >> i;
first.setLength(f, i);
cout << "Enter another distance in feet and inches: ";
cin >> f >> i;
second.setLength(f, i);
// Test the + and - operators
third = first + second;
cout << "first + second = ";
cout << third.getFeet() << " feet, ";
cout << third.getInches() << " inches.\n";
third = first - second;
cout << "first - second = ";
cout << third.getFeet() << " feet, ";
cout << third.getInches() << " inches.\n";
// Test the relational operators
cout << "first == second = ";
if (first == second) cout << "true"; else cout << "false";
cout << "\n";
cout << "first < second = ";
if (first < second) cout << "true"; else cout << "false";
cout << "\n";
return 0;
}
Length.cpp:
#include "Length.h"
//*************************************
// Overloaded operator + *
//*************************************
Length operator+(Length a, Length b)
{
return Length(a.len_inches + b.len_inches);
}
//*************************************
// Overloaded operator - *
//*************************************
Length operator-(Length a, Length b)
{
return Length(a.len_inches - b.len_inches);
}
//************************************
// Overloaded operator == *
//************************************
bool operator==(Length a, Length b)
{
return a.len_inches == b.len_inches;
}
//************************************
// Overloaded operator < *
//************************************
bool operator<(Length a, Length b)
{
return a.len_inches < b.len_inches;
}
Length.h:
#ifndef _LENGTH_H
#define _LENGTH_H
#include <iostream>
using namespace std;
class Length
{
private:
int len_inches;
public:
Length(int feet, int inches)
{
setLength(feet, inches);
}
Length(int inches){ len_inches = inches; }
int getFeet() const { return len_inches / 12; }
int getInches() const { return len_inches % 12; }
void setLength(int feet, int inches)
{
len_inches = 12 *feet + inches;
}
friend Length operator+(Length a, Length b);
friend Length operator-(Length a, Length b);
friend bool operator< (Length a, Length b);
friend bool operator== (Length a, Length b);
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

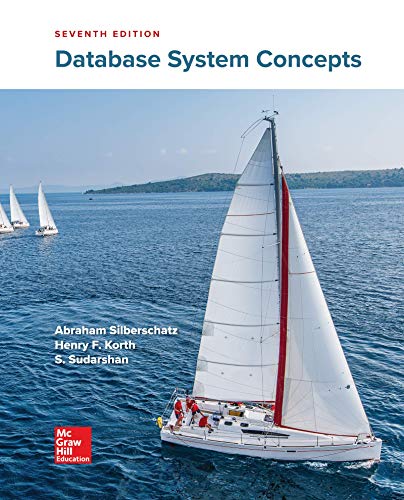
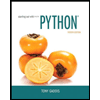
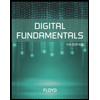
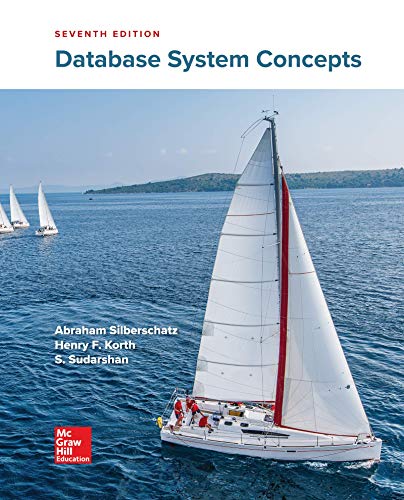
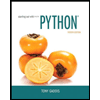
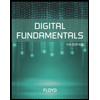
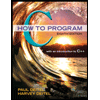
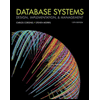
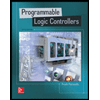