public class DayProg public static void main(String[] args) { // Step 2: Construct a Day object representing today // and assign it to a variable called aDay Date aDay = new Date(); // Step 3: Construct a Day object representing the day for our Exam1 on March 1, 2022, and assign it to a variable called examOne // 22 // 23 Date examone new Date(2022,03,1); 24 // Step 4: Declare three integer variables called 25 year, month and day with initial values of 2022, 4, 12. 26 // // 27 int year=2022, month=4, day=12; 28 29 30 // Step 5: Construct a Day object using the three variables and assign it to a variable called examTwo 31 32 // Date examTwo = new Date(2022,04,12); 33 34 35 // Step 6: Display the three days on separate lines without any messages 36 37 System.out.println(aDay.getYear() +""+aDay.getMonth() System.out.println(examOne.getYear()+""+examOne.getMonth()+""+examOne.getDay()); System.out.println(examTwo.getYear ()+""+examTwo.getMonth()+""+examTwo.getDay )); 38 "+aDay.getDay0); 39 40 41 // Step 7: Display the number of days as a positive integer between the two exams with a message "Number of days between the two exams : 42 // 43 // 44 45 46
public class DayProg public static void main(String[] args) { // Step 2: Construct a Day object representing today // and assign it to a variable called aDay Date aDay = new Date(); // Step 3: Construct a Day object representing the day for our Exam1 on March 1, 2022, and assign it to a variable called examOne // 22 // 23 Date examone new Date(2022,03,1); 24 // Step 4: Declare three integer variables called 25 year, month and day with initial values of 2022, 4, 12. 26 // // 27 int year=2022, month=4, day=12; 28 29 30 // Step 5: Construct a Day object using the three variables and assign it to a variable called examTwo 31 32 // Date examTwo = new Date(2022,04,12); 33 34 35 // Step 6: Display the three days on separate lines without any messages 36 37 System.out.println(aDay.getYear() +""+aDay.getMonth() System.out.println(examOne.getYear()+""+examOne.getMonth()+""+examOne.getDay()); System.out.println(examTwo.getYear ()+""+examTwo.getMonth()+""+examTwo.getDay )); 38 "+aDay.getDay0); 39 40 41 // Step 7: Display the number of days as a positive integer between the two exams with a message "Number of days between the two exams : 42 // 43 // 44 45 46
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![12 public class DayProg
13
public static void main(String[] args)
{
// Step 2: Construct a Day object representing today
14
15
16
//
and assign it to a variable called aDay
17
Date aDay = new Date();
18
19
// Step 3: Construct a Day object representing the day
for our Exam1 on March 1, 2022, and assign
it to a variable called examOne
20
21
//
22
//
Date examOne = new Date(2022,03,1);
23
24
I.
// Step 4: Declare three*integer variables called
25
//
year, month and day with initial values
of 2022, 4, 12.
//
27
int year=2022, month=4, day=12;
28
30
// Step 5: Construct a Day object using the three variables
//
Date examTwo = new Date(2022,04,12);
31
32
and assign it to a variable called examTwo
33
34
35
// Step 6: Display the three days on separate lines
//
System.out.println(aDay.getYear() +""+aDay.getMonth() +""+aDay.getDay ());
System.out.println(examOne.getYear()+""+examOne.getMonth()+""+examOne.getDay());
System.out.println(examTwo .getYear()+""+examTwo.getMonth()+""+examTwo.getDay ());
36
without any messages
39
40
41
//Step 7: Display the number of days as a positive integer
//
between the two exams with a message
43
44
//
"Number of days between the two exams:
MacBook Air
esc
80
888
F1
F2
F3
F4
F5
F6
F7
@
#3
2$
%
&
*
1
2
8.
Q
W
E
R
tab
Y
A
S
F
GI H
caps lock
< cO
3.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F170cb9b5-2292-4ecc-9f24-3c60291ff66d%2F24e0239d-c52b-45ce-9267-e85765c909fb%2F6efdopc_processed.jpeg&w=3840&q=75)
Transcribed Image Text:12 public class DayProg
13
public static void main(String[] args)
{
// Step 2: Construct a Day object representing today
14
15
16
//
and assign it to a variable called aDay
17
Date aDay = new Date();
18
19
// Step 3: Construct a Day object representing the day
for our Exam1 on March 1, 2022, and assign
it to a variable called examOne
20
21
//
22
//
Date examOne = new Date(2022,03,1);
23
24
I.
// Step 4: Declare three*integer variables called
25
//
year, month and day with initial values
of 2022, 4, 12.
//
27
int year=2022, month=4, day=12;
28
30
// Step 5: Construct a Day object using the three variables
//
Date examTwo = new Date(2022,04,12);
31
32
and assign it to a variable called examTwo
33
34
35
// Step 6: Display the three days on separate lines
//
System.out.println(aDay.getYear() +""+aDay.getMonth() +""+aDay.getDay ());
System.out.println(examOne.getYear()+""+examOne.getMonth()+""+examOne.getDay());
System.out.println(examTwo .getYear()+""+examTwo.getMonth()+""+examTwo.getDay ());
36
without any messages
39
40
41
//Step 7: Display the number of days as a positive integer
//
between the two exams with a message
43
44
//
"Number of days between the two exams:
MacBook Air
esc
80
888
F1
F2
F3
F4
F5
F6
F7
@
#3
2$
%
&
*
1
2
8.
Q
W
E
R
tab
Y
A
S
F
GI H
caps lock
< cO
3.

Transcribed Image Text:int year=2022, month=4, day=123;
// Step 5: Construct a Day object using the three variables
and assign it to a variable called examTwo
//
Date examTwo = new Date(2022,04,12);
// Step 6: Display the three days on separate lines
without any messages
//
System.out.println(aDay.getYear() +""+aDay.getMonth() +" "+aDay.getDay(O);
System.out.println(examOne.getYear()+""+examOne.getMonth()+""+examOne.getDay());
System.out.println(examTwo.getYear()+""+examTwo.getMonth()+""+examTwo.getDay());
// Step 7: Display the number of days as a positive integer
between the two exams with a message
"Number of days between the two exams:
// Step 8: Update aDay to the day two days later
Day aDay = new Day();
Day.addDays(7);
System.out.println(aDay.toString());
// Step 9: Print the year, month and day of aDay,
one value per line without any messages
56
57
System.out.println(aDay.getYear());
System.out.println(aDay.getMonth());
System.out.println(aDay.getDay());
MacBook Air
80
88
DII
esc
F1
F2
F3
F4
F5
F7
F8
!
@
#
$
%
&
*
1
3
4
6.
7
8
Q
W
E
Y
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
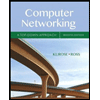
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
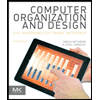
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
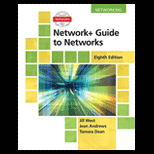
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
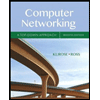
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
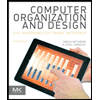
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
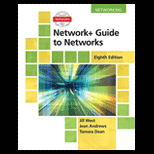
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
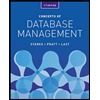
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
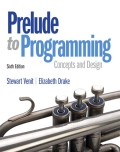
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
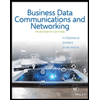
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY