Put the class definition in Temperature.h and the implementation of the constructors and functions in Temperature.cpp 1. Define a class called "Temperature", that represents the information of a temperature. A Temperature is defined with these attributes: value (double), type (char) and city (string). Functions of the Temperature class must perform the following operations: 1. A default constructor which sets all the numeric instance variables to zera, the type to "C" and the String instance variables to empty String. 2. A constructor with 3 parameters which sets the 3 instance variables to the corresponding values passed. 3. Implement a getter function for each of the 3 instance variables that will return the value of the instance variable. For example, the getX() function for the instance variable type must be called get Type(). Implement a setter function for each instance variable that will assign to the instance variable to the value passed. For example, the setX() function for the instance variable type must be called set Type(). 4 5. A Convert function: This function converts the temperature from Fahrenheit to Celsius and from Celsius to Fahrenheit and returns the result. If the user enters 'F' for the type and the temperature value in Fahrenheit, then this function must return the Celsius. As same if user enters 'C' for the type and the temperature value in Centigrade, it must return the Fahrenheit value. Using the two equations below: Fahrenheit Celsius 1.8 +32 Celsius- (Fahrenheit - 32) /1.8 Equation 1 Equation 2 6 An Info function: this function prints the information of the object as following format: o Temperature of +(city)+ "sF="+ [Fahrenheit] + "C=" + (Celsius) 7. A Compare function: This function has one input parameter which is a pointer to a Temperature object and prints a message according to following criteria: of the temperature value of first object (the object that the Compare function is called from) is greater than second object (input parameter in Compare, the program prints: info of first object (using info function)+ new line+ "s greater than"+ new line + print info of second object. of the temperature value of first object is less than second object, the program prints: info of first object (using info function) new line is less than+new line+print info of second object.
Put the class definition in Temperature.h and the implementation of the constructors and functions in Temperature.cpp 1. Define a class called "Temperature", that represents the information of a temperature. A Temperature is defined with these attributes: value (double), type (char) and city (string). Functions of the Temperature class must perform the following operations: 1. A default constructor which sets all the numeric instance variables to zera, the type to "C" and the String instance variables to empty String. 2. A constructor with 3 parameters which sets the 3 instance variables to the corresponding values passed. 3. Implement a getter function for each of the 3 instance variables that will return the value of the instance variable. For example, the getX() function for the instance variable type must be called get Type(). Implement a setter function for each instance variable that will assign to the instance variable to the value passed. For example, the setX() function for the instance variable type must be called set Type(). 4 5. A Convert function: This function converts the temperature from Fahrenheit to Celsius and from Celsius to Fahrenheit and returns the result. If the user enters 'F' for the type and the temperature value in Fahrenheit, then this function must return the Celsius. As same if user enters 'C' for the type and the temperature value in Centigrade, it must return the Fahrenheit value. Using the two equations below: Fahrenheit Celsius 1.8 +32 Celsius- (Fahrenheit - 32) /1.8 Equation 1 Equation 2 6 An Info function: this function prints the information of the object as following format: o Temperature of +(city)+ "sF="+ [Fahrenheit] + "C=" + (Celsius) 7. A Compare function: This function has one input parameter which is a pointer to a Temperature object and prints a message according to following criteria: of the temperature value of first object (the object that the Compare function is called from) is greater than second object (input parameter in Compare, the program prints: info of first object (using info function)+ new line+ "s greater than"+ new line + print info of second object. of the temperature value of first object is less than second object, the program prints: info of first object (using info function) new line is less than+new line+print info of second object.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please write this C++ code by following all the instructions
(2nd image shows the required output of the program ,green coloured is the user's input)
Thank you for your help!
![dnesday [Read-Only]- Microsof
Driver Class (Temperature driver.cpp)
Create a file Temperature driver.cpp with the main function that asks the user to
of two Temperature objects and it dynamically creates two objects of type Temperature. The
Convert, Info and Compare functions, the following results are displayed: (Text in green is
input)
Load first Temperature
Please enter city name: Aden.
What is the unit of Temperature? (F for Fahrenheit, C for Centigrade) C
You choose Celsius, so please enter Celsius value: 30.
Load second Temperature
Please enter city name: Montreal.
What is the unit of Temperature? (F for Fahrenheit, C for Centigrade) F
You choose Fahrenheit, so please enter Fahrenheit value: 12.J
Temperature of Aden is F = 86 C = 30
is greater than
Temperature of Montreal is F = 12 C = -11.11
Load first Temperature
Please enter city name: Ottawa.
What is the unit of Temperature? (F for Fahrenheit, C for Centigrade) F.J
You choose Fahrenheit, so please enter Fahrenheit value: 45
Load second Temperature
Please enter city name: London.
What is the unit of Temperature? (F for Fahrenheit, C for Centigrade) C
You choose Celsius, so please enter Celsius value: 32.J
Temperature of Ottawa is F = 45 C = 7.22
is less than
Temperature of London is F = 89.6 C = 32
Note 1: You are to expect a perfect user who will always enter valid values; that is, do not verify the
validity of user input.
Note 2: Please note that you are supposed to use the pointers at different parts of the lab question. If
you use different methods, even if your program works you do not get any mark.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd5ee8f6f-b196-4b10-99ae-82f5314436a3%2F35f3b13e-7648-410e-985c-9ba7501c0ffc%2Fxfhokyu_processed.jpeg&w=3840&q=75)
Transcribed Image Text:dnesday [Read-Only]- Microsof
Driver Class (Temperature driver.cpp)
Create a file Temperature driver.cpp with the main function that asks the user to
of two Temperature objects and it dynamically creates two objects of type Temperature. The
Convert, Info and Compare functions, the following results are displayed: (Text in green is
input)
Load first Temperature
Please enter city name: Aden.
What is the unit of Temperature? (F for Fahrenheit, C for Centigrade) C
You choose Celsius, so please enter Celsius value: 30.
Load second Temperature
Please enter city name: Montreal.
What is the unit of Temperature? (F for Fahrenheit, C for Centigrade) F
You choose Fahrenheit, so please enter Fahrenheit value: 12.J
Temperature of Aden is F = 86 C = 30
is greater than
Temperature of Montreal is F = 12 C = -11.11
Load first Temperature
Please enter city name: Ottawa.
What is the unit of Temperature? (F for Fahrenheit, C for Centigrade) F.J
You choose Fahrenheit, so please enter Fahrenheit value: 45
Load second Temperature
Please enter city name: London.
What is the unit of Temperature? (F for Fahrenheit, C for Centigrade) C
You choose Celsius, so please enter Celsius value: 32.J
Temperature of Ottawa is F = 45 C = 7.22
is less than
Temperature of London is F = 89.6 C = 32
Note 1: You are to expect a perfect user who will always enter valid values; that is, do not verify the
validity of user input.
Note 2: Please note that you are supposed to use the pointers at different parts of the lab question. If
you use different methods, even if your program works you do not get any mark.
![Put the class definition in Temperature.h and the implementation of the constructors and functions in
Temperature.cpp
1. Define a class called "Temperature", that represents the information of a temperature. A Temperature is
defined with these attributes: value (double), type (char) and city (string). Functions of the Temperature
class must perform the following operations:
1. A default constructor which sets all the numeric instance variables to zero, the type to C and the String
instance variables to empty String.
2.
3.
A constructor with 3 parameters which sets the 3 instance variables to the corresponding values passed.
Implement a getter function for each of the 3 instance variables that will return the value of the instance
variable. For example, the getX() function for the instance variable type must be called get Type().
Implement a setter function for each instance variable that will assign to the instance variable to the
value passed. For example, the setX() function for the instance variable type must be called
set Type().
4.
5. A Convert function: This function converts the temperature from Fahrenheit to Celsius and
from Celsius to Fahrenheit and returns the result of the user enters 'F' for the type and the
temperature value in Fahrenheit, then this function must return the Celsius. As same if user enters 'C'
for the type and the temperature value in Centigrade, it must return the Fahrenheit value. Using the
two equations below:
Fahrenheit Celsius 1.8 +32
Celsius-(Fahrenheit - 32) /1.8
Equation 1
Equation 2
6 An Info function: this function prints the information of the object as following format:
o "Temperature of + (city)+ "isF=" + [Fahrenheit] + "C=" + (Celsius)
7. A Compare function: This function has one input parameter which is a pointer to a Temperature object
and prints a message according to following criteria:
of the temperature value of first object (the object that the Compore function is called from) is
greater than second object (input parameter in Compare, the program prints:
info of first object (using info function)+ new line+ "is greater than new line+ print info of
second object,
D
if the temperature value of first object is less than second object, the program prints
info of first object (using info function) new line+ "s less than"+new line + print info of
second object.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd5ee8f6f-b196-4b10-99ae-82f5314436a3%2F35f3b13e-7648-410e-985c-9ba7501c0ffc%2F5ztnqt5i_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Put the class definition in Temperature.h and the implementation of the constructors and functions in
Temperature.cpp
1. Define a class called "Temperature", that represents the information of a temperature. A Temperature is
defined with these attributes: value (double), type (char) and city (string). Functions of the Temperature
class must perform the following operations:
1. A default constructor which sets all the numeric instance variables to zero, the type to C and the String
instance variables to empty String.
2.
3.
A constructor with 3 parameters which sets the 3 instance variables to the corresponding values passed.
Implement a getter function for each of the 3 instance variables that will return the value of the instance
variable. For example, the getX() function for the instance variable type must be called get Type().
Implement a setter function for each instance variable that will assign to the instance variable to the
value passed. For example, the setX() function for the instance variable type must be called
set Type().
4.
5. A Convert function: This function converts the temperature from Fahrenheit to Celsius and
from Celsius to Fahrenheit and returns the result of the user enters 'F' for the type and the
temperature value in Fahrenheit, then this function must return the Celsius. As same if user enters 'C'
for the type and the temperature value in Centigrade, it must return the Fahrenheit value. Using the
two equations below:
Fahrenheit Celsius 1.8 +32
Celsius-(Fahrenheit - 32) /1.8
Equation 1
Equation 2
6 An Info function: this function prints the information of the object as following format:
o "Temperature of + (city)+ "isF=" + [Fahrenheit] + "C=" + (Celsius)
7. A Compare function: This function has one input parameter which is a pointer to a Temperature object
and prints a message according to following criteria:
of the temperature value of first object (the object that the Compore function is called from) is
greater than second object (input parameter in Compare, the program prints:
info of first object (using info function)+ new line+ "is greater than new line+ print info of
second object,
D
if the temperature value of first object is less than second object, the program prints
info of first object (using info function) new line+ "s less than"+new line + print info of
second object.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
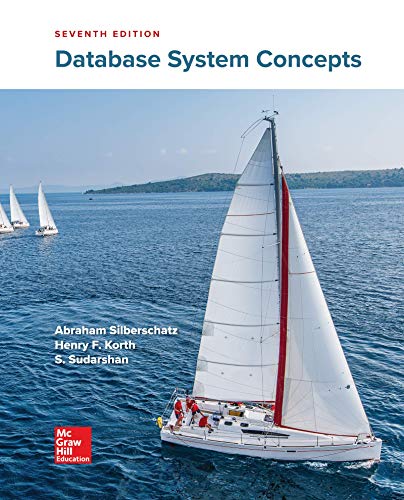
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
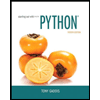
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
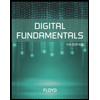
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
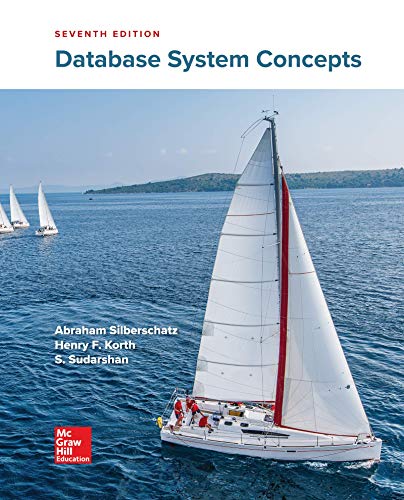
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
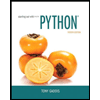
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
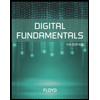
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
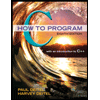
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
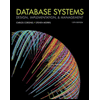
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
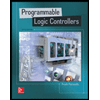
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education