Task 2- Adjacent element swap for a list (Topics: list, loops, functions, string manipulation, assert) The user will input a string as a series of characters separated by spaces. The input string should be converted to a list of characters. Task 2 is to swap the values of every two items in the list; for example – items at positions 0 and 1 are swapped; items 2 and 3 are swapped; items 4 and 5 are swapped, and so on, until the end of the list. You can assume that the list has at least two items – if not, your swap function should raise an AssertionError (see details below). Note that there are two cases: (1) the list has an even number of elements; (2) the list has an odd number of elements. For case 1, swap all elements. For case 2, swap all elements but leave the last element unchanged. See the diagram below for examples. Case 1- even number of elements Case 2- odd number of elements initial list 2 3 initial list 6 7 8 9 10 'W' 'o' O 1 1 2 3 4 5 4 5 'b' 'c' 'd' 'H' 'e' '1' 'l' 'o' '1' 'd' O 1 2 3 4 5 'b' 'a' 'd' 'c' 'f' 'e O 1 2 3 4 5 6 7 8 9 10 'e' 'H' 'l' 'l' ' ' 'o' 'W' '1' 'r' 'd' after adjacency element swap after adjacency element swap Last element is unchanged. Require function In addition to the task2() function, you need to implement the following function: swapAdjacentElements(params: alist) -> no return The parameter alist is a list of characters. The function should modify the list as described above. Note that you should modify the list passed to the function and not create a new list. The function has no return. ASSERT: This function should assert with the following error if the list has less than two (2) characters: "Must enter two or more characters!" Task 2 should behave as follows: (1) Prompt the user to input a sequence of two or more characters separated by spaces. (2) Convert the input string into a list of characters. (3) Print the list. (4) Print the list as a string with no spaces between the characters. (5) Call function swapAdjacentElements() to modify the list. See instructions above. This function does not create a new list; instead, it should modify the list passed as an argument. (6) Print the list again to show that the list has been modified. (7) Print the list as a string again with no spaces between the characters.
Task 2- Adjacent element swap for a list (Topics: list, loops, functions, string manipulation, assert) The user will input a string as a series of characters separated by spaces. The input string should be converted to a list of characters. Task 2 is to swap the values of every two items in the list; for example – items at positions 0 and 1 are swapped; items 2 and 3 are swapped; items 4 and 5 are swapped, and so on, until the end of the list. You can assume that the list has at least two items – if not, your swap function should raise an AssertionError (see details below). Note that there are two cases: (1) the list has an even number of elements; (2) the list has an odd number of elements. For case 1, swap all elements. For case 2, swap all elements but leave the last element unchanged. See the diagram below for examples. Case 1- even number of elements Case 2- odd number of elements initial list 2 3 initial list 6 7 8 9 10 'W' 'o' O 1 1 2 3 4 5 4 5 'b' 'c' 'd' 'H' 'e' '1' 'l' 'o' '1' 'd' O 1 2 3 4 5 'b' 'a' 'd' 'c' 'f' 'e O 1 2 3 4 5 6 7 8 9 10 'e' 'H' 'l' 'l' ' ' 'o' 'W' '1' 'r' 'd' after adjacency element swap after adjacency element swap Last element is unchanged. Require function In addition to the task2() function, you need to implement the following function: swapAdjacentElements(params: alist) -> no return The parameter alist is a list of characters. The function should modify the list as described above. Note that you should modify the list passed to the function and not create a new list. The function has no return. ASSERT: This function should assert with the following error if the list has less than two (2) characters: "Must enter two or more characters!" Task 2 should behave as follows: (1) Prompt the user to input a sequence of two or more characters separated by spaces. (2) Convert the input string into a list of characters. (3) Print the list. (4) Print the list as a string with no spaces between the characters. (5) Call function swapAdjacentElements() to modify the list. See instructions above. This function does not create a new list; instead, it should modify the list passed as an argument. (6) Print the list again to show that the list has been modified. (7) Print the list as a string again with no spaces between the characters.
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 2PE
Related questions
Question
In Python please

Transcribed Image Text:Task 2- Adjacent element swap for a list (Topics: list, loops, functions, string manipulation, assert)
The user will input a string as a series of characters separated by spaces. The input string should be converted to a lst of
characters. Task 2 is to swap the values of every two items in the list; for example – items at positions 0 and 1 are
swapped; items 2 and 3 are swapped; items 4 and 5 are swapped, and so on, until the end of the list. You can assume
that the list has at least two items – if not, your swap function should raise an AssertionError (see details below).
Note that there are two cases: (1) the list has an even number of elements; (2) the list has an odd number of elements.
For case 1, swap all elements. For case 2, swap all elements but leave the last element unchanged.
See the diagram below for examples.
Case 1- even number of elements
Case 2- odd number of elements
initial list
initial list
1
2
3
4.
5
1
2
4
5
6
7
8.
9.
10
'b' 'c' 'd'
'f'
'H'
'e' '1' '1' 'o''' 'W' 'o'
'd'
e'
O 1 2
'b' 'a' 'd' 'c' 'f' 'e'
O 1
ינ 'Hי 'e'
3
4
5
2
3
4
5
6
8
10
'o' 'W' '1' 'r' 'd'
after adjacency element swap
after adjacency element swap
Last element
is unchanged.
Require function
In addition to the task2() function, you need to implement the following function:
swapAdjacentElements (params: alist) -> no return
The parameter alist is a list of characters. The function should modify the list as described above. Note that you
should modify the list passed to the function and not create a new list. The function has no return.
ASSERT: This function should assert with the following error if the list has less than two (2) characters:
"Must enter two or more characters!"
Task 2 should behave as follows:
(1) Prompt the user to input a sequence of two or more characters separated by spaces.
(2) Convert the input string into a list of characters.
(3) Print the list.
(4) Print the list as a string with no spaces between the characters.
(5) Call function swapAdjacentElements() to modify the list.
See instructions above. This function does not create a new list; instead, it should modify the
list passed as an argument.
(6) Print the list again to show that the list has been modified.
(7) Print the list as a string again with no spaces between the characters.
See next page for example output.
![Example of task 2 running (user input in red) – see video link for more examples.
Task2: Swap Adjacent List Elements
Input two or more chars separated by spaces: a bab abab
-----
---- -
Initial list
Example of case 1 (even # of items)
['a', 'b', 'a', 'b', 'a', 'b', 'a', 'b']
String version:
Modified list
'abababab'
['b', 'a', 'b', 'a', 'b', 'a', 'b', 'a']*
String version: 'babababa'
Task2: Swap Adjacent List Elements
---- --
------
Input two or more chars separated by spaces: thisis atest
Initial list
['t', 'h', 'i', 's', 'i', 's', 'a', 't', 'e', 's', 't']
String version: 'thisisatest'
Example of case 2 (odd # of items)
Modified list
['h', 't', 's', 'i', 's', 'i', 't',
String version: 'htsisitaset'
s', 'e', 't']
Task2: Swap Adjacent List Elements
Input two or more chars separated by spaces: 1 2 3 4 5
-------
--- ----
Initial list
['1', '2', '3', '4', '5']
String version: '12345'
Modified list
['2', '1', '4', '3', '5']
String version: '21435'
-------- Task2: Swap Adjacent List Elements
Input two or more chars separated by spaces: 1
--- ---
Example of that causes an
Initial list
AssertionError
['1']
String version: '1'
Modified list
Traceback (most recent call last):
File "final.py", line 119, in <module>
main()
File "final.py", line 11, in main
task2()
File "Cfinal.py", line 71, in task2
swapAdjancentElements (theList)
File "final.py", line 55, in swapAdjancentElements
assert len(thelist) >= 2, "Error"
AssertionError: Error](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbeb43acd-4bfb-4c49-84a8-7d6db7b72498%2Fc8027436-2b6b-45fa-84e4-73f1acb75451%2F5j3rzl_processed.png&w=3840&q=75)
Transcribed Image Text:Example of task 2 running (user input in red) – see video link for more examples.
Task2: Swap Adjacent List Elements
Input two or more chars separated by spaces: a bab abab
-----
---- -
Initial list
Example of case 1 (even # of items)
['a', 'b', 'a', 'b', 'a', 'b', 'a', 'b']
String version:
Modified list
'abababab'
['b', 'a', 'b', 'a', 'b', 'a', 'b', 'a']*
String version: 'babababa'
Task2: Swap Adjacent List Elements
---- --
------
Input two or more chars separated by spaces: thisis atest
Initial list
['t', 'h', 'i', 's', 'i', 's', 'a', 't', 'e', 's', 't']
String version: 'thisisatest'
Example of case 2 (odd # of items)
Modified list
['h', 't', 's', 'i', 's', 'i', 't',
String version: 'htsisitaset'
s', 'e', 't']
Task2: Swap Adjacent List Elements
Input two or more chars separated by spaces: 1 2 3 4 5
-------
--- ----
Initial list
['1', '2', '3', '4', '5']
String version: '12345'
Modified list
['2', '1', '4', '3', '5']
String version: '21435'
-------- Task2: Swap Adjacent List Elements
Input two or more chars separated by spaces: 1
--- ---
Example of that causes an
Initial list
AssertionError
['1']
String version: '1'
Modified list
Traceback (most recent call last):
File "final.py", line 119, in <module>
main()
File "final.py", line 11, in main
task2()
File "Cfinal.py", line 71, in task2
swapAdjancentElements (theList)
File "final.py", line 55, in swapAdjancentElements
assert len(thelist) >= 2, "Error"
AssertionError: Error
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
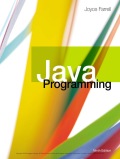
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
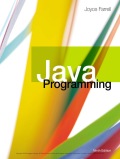
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT