C PROGRAMMING HELP I'm trying to get a portion of my script to work as a udf inside of a header file that already contains two other udfs that are working properly. Here is the script with // lines saying the start and end of the portion i want to be a udf in the header file that I will also paste below the script. Script: #include #include #include "PROJECT HEADER2.h" int main() { // Open the input file FILE *input_file = fopen("Data.txt", "r"); if (input_file == NULL) { printf("Error: could not open input file\n"); return 1; } // Open the output file FILE *output_file = fopen("Result.txt", "w"); if (output_file == NULL) { printf("Error: could not open output file\n"); fclose(input_file); return 1; } //EVERYTHING STARTING HERE // Read the input file and compute coefficients for each line int line_count = 0; double a1, a2, y0, y_prime0, lambda1, lambda2, C1, C2, C_alpha, theta; while (fscanf(input_file, "%lf %lf %lf %lf", &a1, &a2, &y0, &y_prime0) == 4) { // Compute the roots of the equation double discriminant = a2 * a2 - 4 * a1; // Complex roots if (discriminant < 0) { lambda1 = -a2 / (2 * a1); lambda2 = sqrt(-discriminant) / (2 * a1); // Repeated real roots } else if (discriminant == 0) { lambda1 = lambda2 = -a2 / (2 * a1); // Distinct real roots } else { lambda1 = (-a2 + sqrt(discriminant)) / (2 * a1); lambda2 = (-a2 - sqrt(discriminant)) / (2 * a1); } //AND ENDING HERE I WANT IN HEADER FILE // Compute coefficients and write output to file based on type of roots compute_coefficients(lambda1, lambda2, &C1, &C2, &C_alpha, &theta); fprintf(output_file, "%d %lf %lf\n", (lambda1 == lambda2) ? 2 : ((fabs(lambda1 - lambda2) < 1e-10) ? 1 : 3), (lambda1 == lambda2) ? C1 : ((fabs(lambda1 - lambda2) < 1e-10) ? C1 : C_alpha), (lambda1 == lambda2) ? C2 : ((fabs(lambda1 - lambda2) < 1e-10) ? C2 : theta) ); display_output(lambda1, lambda2, C1, C2, C_alpha, theta); line_count++; } // Close the input and output files fclose(input_file); fclose(output_file); return 0; } Header File: #ifndef PROJECT_HEADER2_H #define PROJECT_HEADER2_H //Calculation function void compute_coefficients(double lambda1, double lambda2, double *C1, double *C2, double *C_alpha, double *theta) { if (lambda1 == lambda2) { // Repeated real roots *C1 = 1; *C2 = 0; *C_alpha = lambda1; *theta = 0; } else if (fabs(lambda1 - lambda2) < 1e-10) { // Distinct real roots (with rounding tolerance) *C1 = 1; *C2 = lambda1; *C_alpha = 0; *theta = 0; } else { // Distinct real roots *C1 = (lambda2 - 1) / (lambda2 - lambda1); *C2 = (1 - lambda1) / (lambda2 - lambda1); *C_alpha = (lambda1 - lambda2) / (lambda2 - 1); *theta = atan2(1 - lambda1, lambda2 - 1); } } //Print function void display_output(double lambda1, double lambda2, double C1, double C2, double C_alpha, double theta) { printf("lambda1 = %lf\n", lambda1); printf("lambda2 = %lf\n", lambda2); printf("C1 = %lf\n", C1); printf("C2 = %lf\n", C2); printf("C_alpha = %lf\n", C_alpha); printf("theta = %lf\n", theta); } #endif
C PROGRAMMING HELP
I'm trying to get a portion of my script to work as a udf inside of a header file that already contains two other udfs that are working properly.
Here is the script with // lines saying the start and end of the portion i want to be a udf in the header file that I will also paste below the script.
Script:
#include <stdio.h>
#include <math.h>
#include "PROJECT HEADER2.h"
int main()
{
// Open the input file
FILE *input_file = fopen("Data.txt", "r");
if (input_file == NULL) {
printf("Error: could not open input file\n");
return 1;
}
// Open the output file
FILE *output_file = fopen("Result.txt", "w");
if (output_file == NULL) {
printf("Error: could not open output file\n");
fclose(input_file);
return 1;
}
//EVERYTHING STARTING HERE
// Read the input file and compute coefficients for each line
int line_count = 0;
double a1, a2, y0, y_prime0, lambda1, lambda2, C1, C2, C_alpha, theta;
while (fscanf(input_file, "%lf %lf %lf %lf", &a1, &a2, &y0, &y_prime0) == 4) {
// Compute the roots of the equation
double discriminant = a2 * a2 - 4 * a1;
// Complex roots
if (discriminant < 0) {
lambda1 = -a2 / (2 * a1);
lambda2 = sqrt(-discriminant) / (2 * a1);
// Repeated real roots
} else if (discriminant == 0) {
lambda1 = lambda2 = -a2 / (2 * a1);
// Distinct real roots
} else {
lambda1 = (-a2 + sqrt(discriminant)) / (2 * a1);
lambda2 = (-a2 - sqrt(discriminant)) / (2 * a1);
}
//AND ENDING HERE I WANT IN HEADER FILE
// Compute coefficients and write output to file based on type of roots
compute_coefficients(lambda1, lambda2, &C1, &C2, &C_alpha, &theta);
fprintf(output_file, "%d %lf %lf\n",
(lambda1 == lambda2) ? 2 : ((fabs(lambda1 - lambda2) < 1e-10) ? 1 : 3),
(lambda1 == lambda2) ? C1 : ((fabs(lambda1 - lambda2) < 1e-10) ? C1 : C_alpha),
(lambda1 == lambda2) ? C2 : ((fabs(lambda1 - lambda2) < 1e-10) ? C2 : theta)
);
display_output(lambda1, lambda2, C1, C2, C_alpha, theta);
line_count++;
}
// Close the input and output files
fclose(input_file);
fclose(output_file);
return 0;
}
Header File:
#ifndef PROJECT_HEADER2_H
#define PROJECT_HEADER2_H
//Calculation function
void compute_coefficients(double lambda1, double lambda2, double *C1, double *C2, double *C_alpha, double *theta)
{
if (lambda1 == lambda2) {
// Repeated real roots
*C1 = 1;
*C2 = 0;
*C_alpha = lambda1;
*theta = 0;
} else if (fabs(lambda1 - lambda2) < 1e-10) {
// Distinct real roots (with rounding tolerance)
*C1 = 1;
*C2 = lambda1;
*C_alpha = 0;
*theta = 0;
} else {
// Distinct real roots
*C1 = (lambda2 - 1) / (lambda2 - lambda1);
*C2 = (1 - lambda1) / (lambda2 - lambda1);
*C_alpha = (lambda1 - lambda2) / (lambda2 - 1);
*theta = atan2(1 - lambda1, lambda2 - 1);
}
}
//Print function
void display_output(double lambda1, double lambda2, double C1, double C2, double C_alpha, double theta)
{
printf("lambda1 = %lf\n", lambda1);
printf("lambda2 = %lf\n", lambda2);
printf("C1 = %lf\n", C1);
printf("C2 = %lf\n", C2);
printf("C_alpha = %lf\n", C_alpha);
printf("theta = %lf\n", theta);
}
#endif
Thank you for your help!

Step by step
Solved in 4 steps

That didn't quite work for me, can you do a demonstration please?
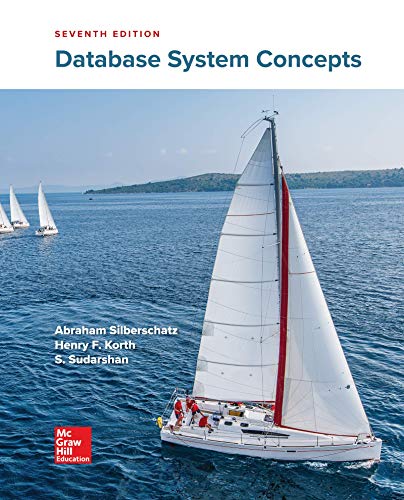
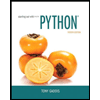
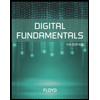
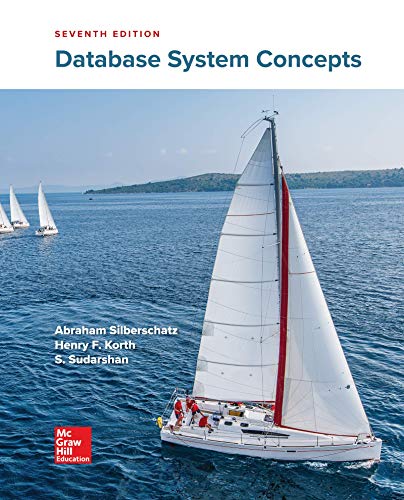
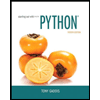
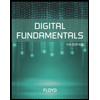
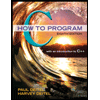
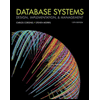
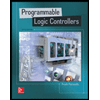